Introduction
The Devastator platform is constructed from high strength aluminum alloy which makes it extremely solid and durable. It is fully compatible with popular microcontrollers on the market, such as Arduino, Raspberry Pi, Lattepanda and so on. The high speed motors and premium quality tracks also allow it to move swiftly over surfaces and its high performance suspension and has outstanding mobility across even the toughest terrains.
The platform itself has multiple mounting holes that allow users to add various sensors, servos, turntables and controllers (e.g. Romeo All-in-one, Raspberry Pi Model B+ etc.) This kit is perfect for hobbyists, educators, robot competitions and research projects. This enhanced kit uses metal geared motors for extra traction and durability.
Possible Applications
- Education
- Competitions
- Mobile robotic arms
- Outdoor 2WD mobile robots
Specification
Working Voltage:3-8V DC
Drive Wheel Diameter: 43mm
Maximum Speed: 36cm/s
Dimensions: 225*220*108
Weight: 780g
Motor Specification: Micro DC metal geared motors x2
Assembly
Chassis
For chassis assembly please refer to the Assembly manual
Electronics
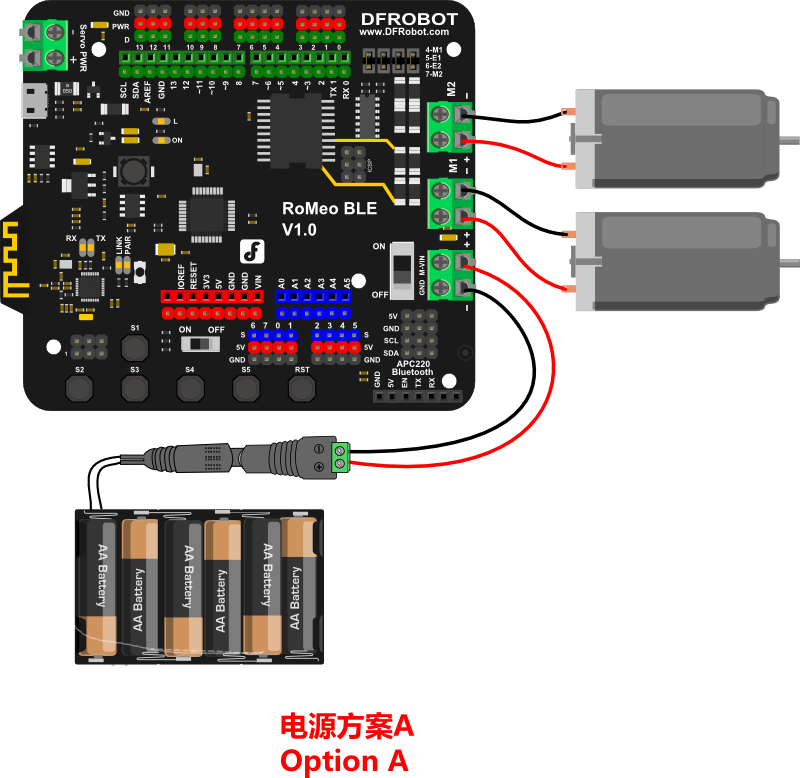
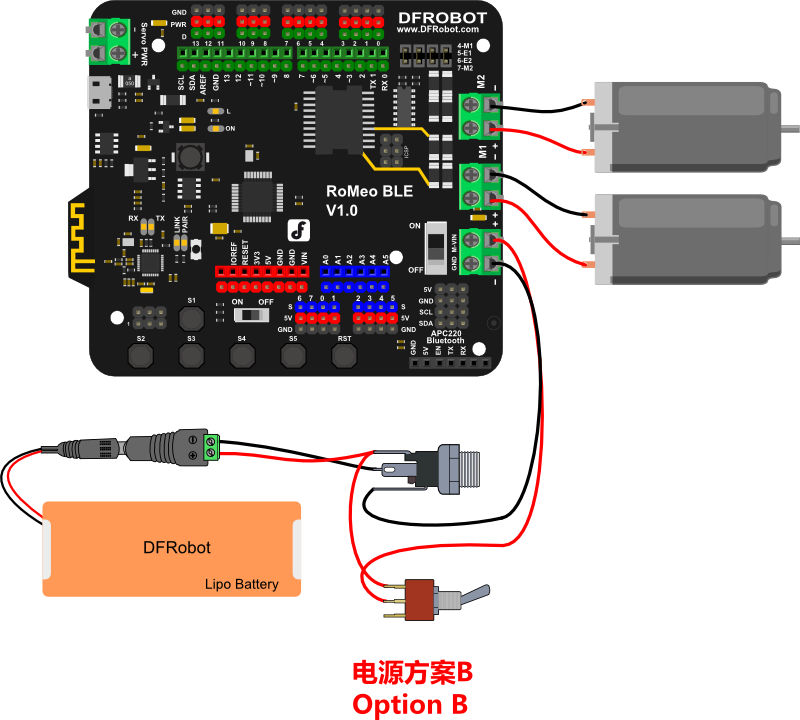
Microcontroller Power Connections
We recommend soldering wires for good electronic continuity. Use a phillips screwdriver to loosen all the motor terminal connections before making a connection.
Take a length of wire and connect it between the positive terminal of the microcontrollers M-VIN terminal and the middle pole of the switch. Take another length of wire and connect it from the left pole of the switch to the positive terminal of the battery charging jack. From the same terminal of the jack, connect another length of wire to the positive terminal of the power supply (or a female jack adapter, as in the diagram).
The GND wire needs to run from the GND terminal of the microcontroller (next to M-VIN terminal). It needs to run to the negative terminal of the battery charger jack, and then from the same negative terminal of the battery charger jack to the negative terminal of the power supply.
Motor Connections
We recommend soldering wires to each motor for good electronic continuity. Tinning the opposite end of the wire is also a good idea. Use a phillips screwdriver to loosen M1's motor terminals on the microcontroller. Insert the positive and negative motor wires in to positive and negative terminals on the microcontroller, and tighten the terminals again. Repeat the process for M2.
Supplying Power
You can power the robot by 6 x AA batteries or a 7.5v LiPo battery. We recommend the LiPo battery option as it is rechargeable.
Sample Code
This sample code will allow keyboard control your Devastator platform.
/*
# Editor : Phoebe
# Date : 2014.11.6
# Ver : 0.1
# Product: Devastator Tank Mobile Platform
# SKU : RBO0112
# Description:
# Connect the D4,D5,D6,D7,GND to UNO digital 4,5,6,7,GND
*/
int E1 = 5; //M1 Speed Control
int E2 = 6; //M2 Speed Control
int M1 = 4; //M1 Direction Control
int M2 = 7; //M1 Direction Control
void stop(void) //Stop
{
digitalWrite(E1,0);
digitalWrite(M1,LOW);
digitalWrite(E2,0);
digitalWrite(M2,LOW);
}
void advance(char a,char b) //Move forward
{
analogWrite (E1,a); //PWM Speed Control
digitalWrite(M1,HIGH);
analogWrite (E2,b);
digitalWrite(M2,HIGH);
}
void back_off (char a,char b) //Move backward
{
analogWrite (E1,a);
digitalWrite(M1,LOW);
analogWrite (E2,b);
digitalWrite(M2,LOW);
}
void turn_L (char a,char b) //Turn Left
{
analogWrite (E1,a);
digitalWrite(M1,LOW);
analogWrite (E2,b);
digitalWrite(M2,HIGH);
}
void turn_R (char a,char b) //Turn Right
{
analogWrite (E1,a);
digitalWrite(M1,HIGH);
analogWrite (E2,b);
digitalWrite(M2,LOW);
}
void setup(void)
{
int i;
for(i=4;i<=7;i )
pinMode(i, OUTPUT);
Serial.begin(19200); //Set Baud Rate
Serial.println("Run keyboard control");
digitalWrite(E1,LOW);
digitalWrite(E2,LOW);
}
void loop(void)
{
if(Serial.available()){
char val = Serial.read();
if(val != -1)
{
switch(val)
{
case 'w'://Move Forward
advance (255,255); //move forward in max speed
break;
case 's'://Move Backward
back_off (255,255); //move back in max speed
break;
case 'a'://Turn Left
turn_L (100,100);
break;
case 'd'://Turn Right
turn_R (100,100);
break;
case 'z':
Serial.println("Hello");
break;
case 'x':
stop();
break;
}
}
else stop();
}
}
Once the code has uploaded, keep the USB cable plugged in. Make sure the Devastator's switch is ON and that a power supply is connected - e.g.: a lipo battery.
Open the Arduno IDE serial monitor. Set the bottom panels to "No line ending" and the baud rate to 9600. This is important as the microcontroller needs to communicate with your computer for this program to work properly.
These are basic instructions for this program. Using the W, D, A, S, and Z keys on your keyboard, try moving the Devastator. When you press "W", the Devastator should move forward When you press "D", the Devastator should turn to the right When you press "A", the Devastator should turn to the left When you press "S", the Devastator should move backwards When you press "Z", the Arduino IDE serial monitor should print: "Hello"
Changing the Motor Direction in Code
Let's examine some of the global variables in the program:
int M1 = 4; //M1 Direction Control
int M2 = 7; //M2 Direction Control
Digital pins 4 and 7 have been assigned as the motor direction control pins. By setting each either HIGH or LOW (i.e. on or off), we can control which way the motor will turn.
Let's examine another section of code:
//TURN LEFT
void turn_L (char a, char b)
{
analogWrite (E1, a);
digitalWrite(M1, LOW);
analogWrite (E2, b);
digitalWrite(M2, HIGH);
}
This is a function that tells the Devastator to turn left. M1 and M2 are set as LOW and HIGH respectively. This means that the left-side wheels will turn backwards and the right-side wheels turn forwards.
Conversely, turning right has the motor pins set like so:
//TURN RIGHT
void turn_R (char a, char b)
{
analogWrite (E1, a);
digitalWrite(M1, HIGH);
analogWrite (E2, b);
digitalWrite(M2, LOW);
}
Therefore, if you find that your Devastator's wheels are going in a direction you don't intend them to, try changing the motor pins signal. If they are going the wrong way and the direction pin is set to HIGH, try changing it to LOW, and vice versa. You can use the keyboard control program to debug and verify these settings.
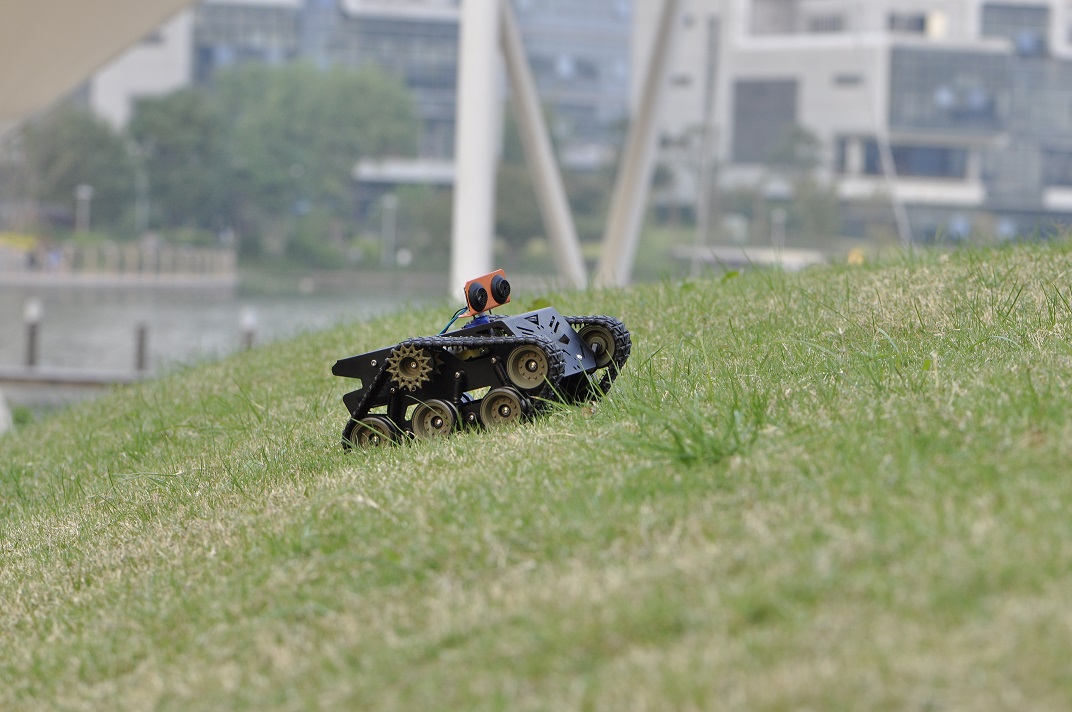
Using GoBLE Control
If you want to control your Devastator using your iPhone as a remote, you can achieve this using GoBLE. More information can be found at the following link: https://www.dfrobot.com/wiki/index.php?title=Bluetooth_APP_Control
The GoBLE library can be found here: https://github.com/CainZ/GoBle/
Here is a sample code that implements GoBLE control:
/*
# Edited: Richard/Matt
# Date: 2016.03.11
# Product: Devastator Tank Mobile Platform DC Motor Metal Gear
# SKU: ROB0128
# GoBLE library available at: https://github.com/CainZ/GoBle/
*/
//include libraries
#include <GoBLE.h>
#include <QueueArray.h>
#include <Servo.h>
#include <Metro.h>
//assign motor pins and direction pins
int speedPin_M1 = 5; //M1 Speed Control
int speedPin_M2 = 6; //Speed Control
int directionPin_M1 = 4; //Direction Control
int directionPin_M2 = 7; //M1 Direction Control
int joystickX, joystickY; //create joystick variables for goble
void setup() { //the setup function runs once
Goble.begin(); //begin GoBLE
Serial.begin(115200); //serial communication speed
}
void loop() { //the main loop runs forever (or until you power off the microcontroller or a component fails)
if (Goble.available()) { //check for a bluetooth connection to goBLE
joystickX = Goble.readJoystickX(); //read joystick x value
joystickY = Goble.readJoystickY(); //read joystick y value
//use the serial monitor to debug
//(x and y joystick values are from 0 - 255)
// Serial.print("Joystick Value: ");
// Serial.print(joystickX);
// Serial.print(" ");
// Serial.println(joystickY);
if (joystickY > 196) { //if joystick y > 196 then turn right at speed 250
Serial.println("turn right");
carTurnRight(250, 250);
}
else if (joystickY < 64) { // //if joystick y < 64 then turn left at speed 250
Serial.println("turn left");
carTurnLeft(250, 250);
}
else if (joystickX > 196) { //if joystick x > 196 then go forward at speed 500
Serial.println("move forward");
carAdvance(500, 500);
}
else if (joystickX < 64) { //if joystick x <64 then go backward at speed 500
Serial.println("move backward");
carBack(500, 500);
}
else {
carStop(); //or else stop
}
}
}
//tank movement functions
void carStop() { // Motor Stop
digitalWrite(speedPin_M2, 0);
digitalWrite(directionPin_M1, LOW);
digitalWrite(speedPin_M1, 0);
digitalWrite(directionPin_M2, LOW);
}
void carTurnLeft(int leftSpeed, int rightSpeed) { //Turn Left
analogWrite (speedPin_M2, leftSpeed); //PWM Speed Control
digitalWrite(directionPin_M1, HIGH);
analogWrite (speedPin_M1, rightSpeed);
digitalWrite(directionPin_M2, LOW);
}
void carTurnRight(int leftSpeed, int rightSpeed) { //Turn Right
analogWrite (speedPin_M2, leftSpeed);
digitalWrite(directionPin_M1, LOW);
analogWrite (speedPin_M1, rightSpeed);
digitalWrite(directionPin_M2, HIGH);
}
void carBack(int leftSpeed, int rightSpeed) { //Move backward
analogWrite (speedPin_M2, leftSpeed);
digitalWrite(directionPin_M1, LOW);
analogWrite (speedPin_M1, rightSpeed);
digitalWrite(directionPin_M2, LOW);
}
void carAdvance(int leftSpeed, int rightSpeed) { //Move forward
analogWrite (speedPin_M2, leftSpeed);
digitalWrite(directionPin_M1, HIGH);
analogWrite (speedPin_M1, rightSpeed);
digitalWrite(directionPin_M2, HIGH);
}
FAQ
Q&A | Some general Arduino Problems/FAQ/Tips |
---|---|
Q | The track is a little loose, is there any way I can do to tight the track? |
A | To change the intension of the track, you just need a strong and fine tool, here I use tweezers. 1. Find the thin bolt used to connect track shoes, normally the side shown on the picture is easier to push ![]() 2. Use the tweezes to push the bolt out of the track, it may be a little tight, be careful! ![]() 3. Remove the bolt, do the same steps to remove some track shoes according to your Devastator ![]() 4. Insert the bolt back and finish! ![]() |
For any questions, advice or cool ideas to share, please visit the DFRobot Forum.
More Documents
Get Devastator Tank Mobile Robot Platform from DFRobot Store or DFRobot Distributor.