Introduction
The VL53L0X range finder is a high-precision distance finder that based on new Time-of-Flight (ToF) principle. VL53L0X provides accurate distance measurement whatever the target reflectance unlike traditional technology. It can measure absolute distances up to 2m.
DFRobot has introduced VL53L0X into Gravity Series. It provides Gravity-I2C interface, plug and play, supports 3.3V~5V power supply, and is compatible with more microcontrollers, and adapt to more application scenarios than others.
The VL53L0X integrates a leading-edge SPAD array (Single Photon Avalanche Diodes) and embeds ST’s second generation FlightSenseTM patented technology. Its accuracy is ±3%, response time is less than 30ms, power consumption is only 20mW in normal operation mode, stand-by power consumption is 5uA.
The VL53L0X's 940nm VCSEL emitter (vertical cavity surface emitting laser) is totally invisible to the human eye, coupled with internal physical infrared filters, it enables longer ranging distance, higher immunity to ambient light and better robustness to cover-glass optical cross-talk.
Specification
- Power supply: 3.3-5V DC
- Operating Voltage: 2.8V
- Infrared emitter: 940nm
- Range: 30-2000mm
- FOV: 25°
- Ranging Accuracy: ±3%
- Sampling Time: <= 30ms
- Operating Temperature: -20-70 °C
- Interface Type: Gravity-I2C
- Product Size: 0.79*0.87 in
Pin Description
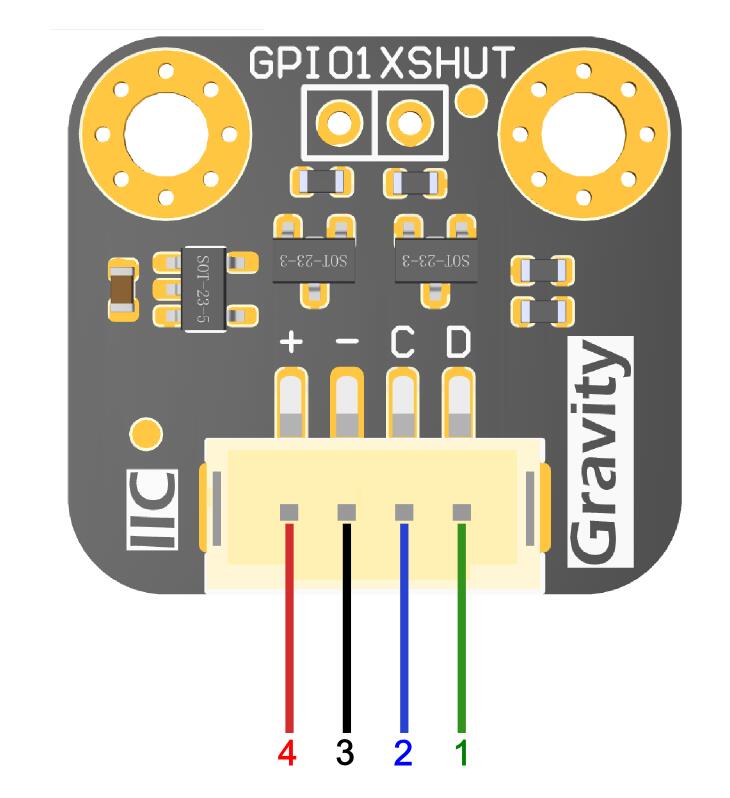
Label | '''Name ''' | Description |
---|---|---|
1 green line | D | DATA |
2 blue line | C | CLK |
3 black line | - | GND |
4 red line | + | VCC |
GPIO1: interrupt output pin to indicate if the data is ready XSHUT: Close the pin of the sensor, pulled high by default, sensor enters off mode when pin is pulled low
Tutorial
Requirements
Hardware
- Arduino UNO control board x1.
- VL53L0X Distance detection Sensor module
Software
- Arduino IDE, Click to Download Arduino IDE from Arduino®
Connection Diagram
Sample Code
/*!
* @file DFRobot_VL53L0X.ino
* @brief DFRobot's Laser rangefinder library
* @n The example shows the usage of VL53L0X in a simple way.
* @copyright [DFRobot](https://www.dfrobot.com), 2016
* @copyright GNU Lesser General Public License
* @author [LiXin]
* @version V1.0
* @date 2017-8-21
* @https://github.com/DFRobot/DFRobot_VL53L0X
timer*/
#include "Arduino.h"
#include "Wire.h"
#include "DFRobot_VL53L0X.h"
/*****************Keywords instruction*****************/
//Continuous--->Continuous measurement model
//Single------->Single measurement mode
//High--------->Accuracy of 0.25 mm
//Low---------->Accuracy of 1 mm
/*****************Function instruction*****************/
//setMode(ModeState mode, PrecisionState precision)
//*This function is used to set the VL53L0X mode
//*mode: Set measurement mode Continuous or Single
//*precision: Set the precision High or Low
//void start()
//*This function is used to enabled VL53L0X
//float getDistance()
//*This function is used to get the distance
//uint16_t getAmbientCount()
//*This function is used to get the ambient count
//uint16_t getSignalCount()
//*This function is used to get the signal count
//uint8_t getStatus();
//*This function is used to get the status
//void stop()
//*This function is used to stop measuring
DFRobotVL53L0X sensor;
void setup() {
//initialize serial communication at 115200 bits per second:
Serial.begin(115200);
//join i2c bus (address optional for master)
Wire.begin();
//Set I2C sub-device address
sensor.begin(0x50);
//Set to Back-to-back mode and high precision mode
sensor.setMode(Continuous,High);
//Laser rangefinder begins to work
sensor.start();
}
void loop()
{
//Get the distance
Serial.print("Distance: ");Serial.println(sensor.getDistance());
//The delay is added to demonstrate the effect, and if you do not add the delay,
//it will not affect the measurement accuracy
delay(500);
}
Expected Results
Measured Results
actual distance | measuring distance(erro) | actual distance | measuring distance(erro) | actual distance | measuring distance(erro) | actual distance | measuring distance(erro) |
---|---|---|---|---|---|---|---|
500 | 490(-10) 491.5(-8.5) 498(-2) 496.5(-3.5) 499.75(-0.25) 503.75(+3.75) |
300 | 304.75(+4.75) 298.75(-1.25) 299.5(-0.5) 302(+2) 297.5(-2.5) 299.5(-0.5) |
138 | 138.5(+0.5) 131.5(-6.5) 132.25(-5.75) 131.25(-6.75) 136(-2) 138.5(+0.5) |
100 | 102(+2) 109(+9) 105.5(+5.5) 104.75(+4.75) 99.5(-0.5) 102.5(+2.5) |
API List
#include <DFRobot_EINK.h>
DFRobotVL53L0X sensor //Create an object
/*
* @Function: set range mode.
* @Parameter 1 mode: ranging mode
* Single: Single range
* Continuous: Continuous range
* @parameter 2 precision: Measuring precision
* High:high precision(0.25mm)
* Low:standard accuracy(1mm)
*/
void setMode(uint8_t mode, uint8_t precision);
/*
* @function:start measuring
*/
void start();
/*
* @function:stop measuring
*/
void stop();
/*
* @function:acquire distance
*/
uint16_t getDistance();
/*
* @function:acquire environmental variables
*/
uint16_t getAmbientCount();
/*
* @function:acquire semaphore
*/
uint16_t getSignalCount();
Compatibility Test
MCU | Pass the test | Failed the test | No test | Note |
---|---|---|---|---|
FireBeetle-Board328P | √ | |||
FireBeetle-ESP32 | √ | |||
FireBeetle-ESP8266 | √ | |||
Leonardo | √ |
Dimension
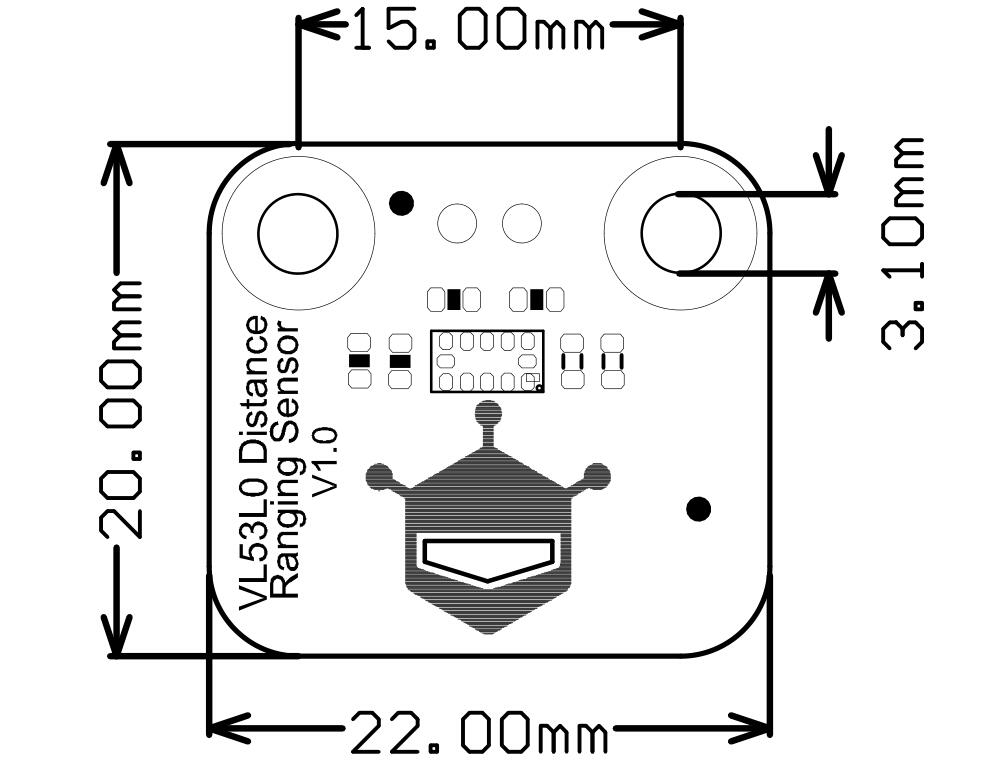
FAQ
For any questions, advice or cool ideas to share, please visit the DFRobot Forum. |
---|