Introduction
Our new Formaldehyde (HCHO) sensor module is Arduino and Raspberry Pi compatible and is an ideal solution for an indoor air quality monitor.
Usually HCHO is measured in air by reagent or colourimetric card, but these solutions are inefficient, can only be used once, and the end result is not always reliable.
The formaldehyde (HCHO) sensor module is compact and fully compatible with Arduino. Through it, you can measure the concentration of formaldehyde in air and measure the air quality. This sensor module is widely used in many air testing applications, such as indoor air quality applications or real time air quality monitoring stations. The HCHO sensor module can detect and measure HCHO with a high level of accuracy. It also has many advantages such as strong anti-jamming capabilities, high stability, ATC, high sensitivity (of up to 0.01ppm) and a long life guarantee (2 years in outdoor conditions). This module uses a 3 pin Gravity Interface for easy plug and play usage. With a wide input voltage range, and optional output signal, our module is compatible with a wide range of microcontrollers, including Arduino and Raspberry Pi. Use our pre-written sample codes to get your HCHO detector up and running very quickly. Used in an IoT application, it is possible to make an automatic air quality station which can measure HCHO in different sites.
![]() |
When using the sensor module, avoid touching the white sensing film. Keep away from high concentration Hydrogen sulfide, hydrogen, methanol, ethanol and carbon monoxide. These gases will affect the precision and reduce the service life. We recommend priming the module by powering it for 5 minutes on first use. Avoid organic solvents, coatings, medicines, oils and high concentration gases Do not plug unplug the sensor on the module, and do not modify components on the PCB. Avoid excessive impact or vibration. Do not use the modules in systems that are related to human being’s safety. Do not use the modules in strong convection environments. Do not expose modules in high concentration organic gas for extended periods of time. |
---|
Specification
- Input Voltage: 3.3~6V
- Target Gas: HCHO (CH2O)
- Interference Gas: Alcohol, CO & etc
- Detection Range: 0~5ppm
- Resolution: 0.01ppm
- Warm Up Time: ≤3 minutes
- Response Time: ≤60 seconds
- Resume Time: ≤60 seconds
- Interface Type: Gravity PH2.0 3Pin
- Output Signal: Optional DAC(0.4~2V,for 0-5ppm) or UART(9600)
- Operating Temp: 0~50℃
- Operating Hum: 15%RH-90%RH(No Condensation)
- Storage Temp: 0~50℃
- Working Life: 2 years(in air)
Board Overview
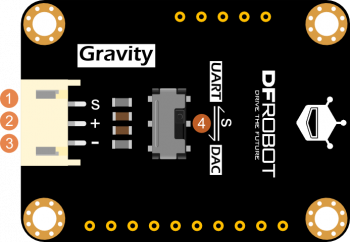
Num | Label | Description |
---|---|---|
1 | S | Signal (DAC/UART) |
2 | + | VCC |
3 | - | GND |
4 | SW | Signal (UART or DAC) Output Switch |
Tutorial
This tutorial will show how to use this HCHO sensor.
The output signal can be UART mode or DAC mode.
![]() |
We recommend to use UART mode for more accurate and stable result. |
---|
Requirements
-
Hardware
- DFRduino UNO (or similar) x 1
- HCHO Sensor Module x 1
- Gravity 3P Cable x 1
-
Software
- Arduino IDE, Click to Download Arduino IDE from Arduino®
UART Mode
Before using UART mode, please shift the switch to UART mode. In this mode, we'll use SoftwareSerial Port to read the data. Here is the data formula.
Connection Diagram
Sample Code
Please install DFRobotHCHOSensor Arduino Library first.
How to install Libraries in Arduino IDE.
/***************************************************
DFRobot Gravity: HCHO Sensor
<https://www.dfrobot.com/wiki/index.php/Gravity:_HCHO_Sensor_SKU:_SEN0231>
***************************************************
This example reads the concentration of HCHO in air by UART mode.
Created 2016-12-15
By Jason <jason.ling@dfrobot.com@dfrobot.com>
GNU Lesser General Public License.
See <http://www.gnu.org/licenses/> for details.
All above must be included in any redistribution
****************************************************/
/***********Notice and Trouble shooting***************
1. This code is tested on Arduino Uno with Arduino IDE 1.0.5 r2.
2. In order to protect the sensor, do not touch the white sensor film on the sensor module,
and high concentration of Hydrogen sulfide, hydrogen, methanol, ethanol, carbon monoxide should be avoided.
3. Please do not use the modules in systems which related to human being’s safety.
****************************************************/
#include <DFRobotHCHOSensor.h>
#include <SoftwareSerial.h>
#define SensorSerialPin 10 //this pin read the uart signal from the HCHO sensor
SoftwareSerial sensorSerial(SensorSerialPin,SensorSerialPin);
DFRobotHCHOSensor hchoSensor(&sensorSerial);
void setup()
{
sensorSerial.begin(9600); //the baudrate of HCHO is 9600
sensorSerial.listen();
Serial.begin(9600);
}
void loop()
{
if(hchoSensor.available()>0)
{
Serial.print(hchoSensor.uartReadPPM());
Serial.println("ppm");
}
}
Expected Results
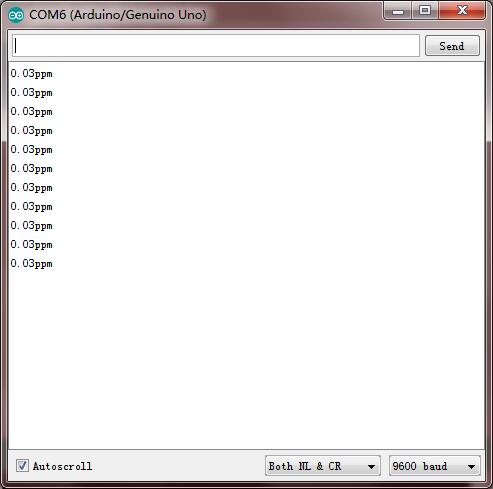
As indicated above, the value will be printed on the serial monitor every second.
DAC Mode
Before using DAC mode, please shift the switch to DAC mode. In this mode, we'll use Arduino Analog port to read the value.
![]() |
In DAC mode, the measurement accuracy will be affected by the bits of the ADC and reference voltage. So the accurate power supply or reference voltage and 10-bit ADC are recommended. |
---|
The relation between voltage(V) and concentration(PPM) are linear.
0.4V -> 0ppm
2.0V -> 5ppm
So the function diagram is shown as the following:
Connection Diagram
Sample Code
Click here to download the sample code first.
/***************************************************
DFRobot Gravity: HCHO Sensor
<https://www.dfrobot.com/wiki/index.php/Gravity:_HCHO_Sensor_SKU:_SEN0231>
***************************************************
This example reads the concentration of HCHO in air by DAC mode.
Created 2016-12-15
By Jason <jason.ling@dfrobot.com@dfrobot.com>
GNU Lesser General Public License.
See <http://www.gnu.org/licenses/> for details.
All above must be included in any redistribution
****************************************************/
/***********Notice and Trouble shooting***************
1. This code is tested on Arduino Uno with Arduino IDE 1.0.5 r2.
2. In order to protect the sensor, do not touch the white sensor film on the sensor module,
and high concentration of Hydrogen sulfide, hydrogen, methanol, ethanol, carbon monoxide should be avoided.
3. Please do not use the modules in systems which related to human being’s safety.
****************************************************/
#define SensorAnalogPin A2 //this pin read the analog voltage from the HCHO sensor
#define VREF 5.0 //voltage on AREF pin
void setup()
{
Serial.begin(9600);
}
void loop()
{
Serial.print(analogReadPPM());
Serial.println("ppm");
delay(1000);
}
float analogReadPPM()
{
float analogVoltage = analogRead(SensorAnalogPin) / 1024.0 * VREF;
float ppm = 3.125 * analogVoltage - 1.25; //linear relationship(0.4V for 0 ppm and 2V for 5ppm)
if(ppm<0) ppm=0;
else if(ppm>5) ppm = 5;
return ppm;
}
Expected Results
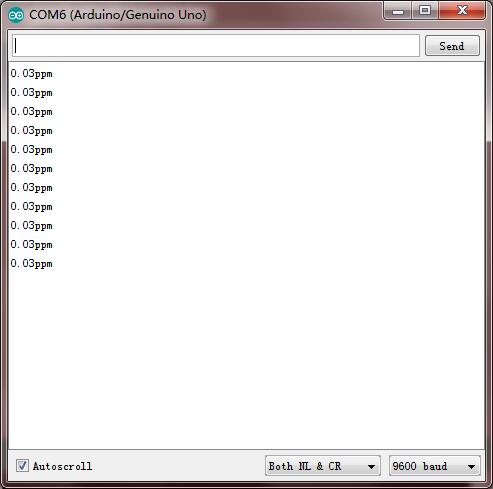
As indicated above, the value will be printed on the serial monitor every second.
FAQ
Q1. There is no data on the UART from sensor when just power on,is it normal? |
---|
A. This sensor module needs some time for Initialization, so it will send signal after a few seconds when power on. |
Q2. What's the relationship between ppm and mg/m3? |
---|
A. In standard condition:1 ppm = 0.746 mg/m3 |
Q3. What's the precision? |
---|
A. 0 - 0.2mg/m3,±0.02mg/m3;> 0.2mg/m3,15% - 20% |
For any questions, advice or cool ideas to share, please visit the DFRobot Forum. |
---|