Introduction
Arduino has a small amount of data storage space, The Arduino UNO has 32k. It doesn't have enough space if you want to store a lot of data. Therefore, we need to add an EEPROM Data Storage Module to increase its storage space. The EEPROM Data Storage Module transmit data to Arduino UNO using I2C Protocol. It used 24LC256 256K I2C CMOS Serial EEPROM provided by Microchip Techonogy Inc. 24LC256 is a 32K x 8(256Kbit) Serial Electrically Erasable Programmable Read - Only Memory. It can also be used with the Interface shield board by I2C on Board.
Specification
- Power Supply:2.5V ~5.5V
- Low-Power CMOS Technology
- Read current: 400 uA max. at 5.5V, 400 kHz
- Standby current: 1 uA max. at 3.6V, I-temp
- Interface: I2C Protocol,2-Wire Serial Interface
- EEPROM: 32K x 8 (256 Kbit)
- Size: 30.00*21.05(mm)
- Mounting Hole Dia: 3.5mm
- Ambient temperature with power applied: -40℃~+125℃
- Weight: 10g
Pin Definition
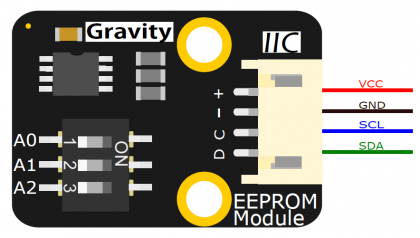
Mark | Name | Function |
---|---|---|
+ | VCC | +2.5V to 5.5V |
- | GND | Ground |
C | SCL | I2C Serial Clock |
D | SDA | I2C Serial Data |
Address True Table
Connection Diagram
Sample Code
/*
* Use the I2C bus with EEPROM 24LC64
* Sketch: eeprom.pde
*
* Author: hkhijhe
* Date: 01/10/2010
*
*
*/
#include <Wire.h> //I2C library
void i2c_eeprom_write_byte( int deviceaddress, unsigned int eeaddress, byte data ) {
int rdata = data;
Wire.beginTransmission(deviceaddress);
Wire.write((int)(eeaddress >> 8)); // MSB
Wire.write((int)(eeaddress & 0xFF)); // LSB
Wire.write(rdata);
Wire.endTransmission();
}
// WARNING: address is a page address, 6-bit end will wrap around
// also, data can be maximum of about 30 bytes, because the Wire library has a buffer of 32 bytes
void i2c_eeprom_write_page( int deviceaddress, unsigned int eeaddresspage, byte* data, byte length ) {
Wire.beginTransmission(deviceaddress);
Wire.write((int)(eeaddresspage >> 8)); // MSB
Wire.write((int)(eeaddresspage & 0xFF)); // LSB
byte c;
for ( c = 0; c < length; c++)
Wire.write(data[c]);
Wire.endTransmission();
}
byte i2c_eeprom_read_byte( int deviceaddress, unsigned int eeaddress ) {
byte rdata = 0xFF;
Wire.beginTransmission(deviceaddress);
Wire.write((int)(eeaddress >> 8)); // MSB
Wire.write((int)(eeaddress & 0xFF)); // LSB
Wire.endTransmission();
Wire.requestFrom(deviceaddress,1);
if (Wire.available()) rdata = Wire.read();
return rdata;
}
// maybe let's not read more than 30 or 32 bytes at a time!
void i2c_eeprom_read_buffer( int deviceaddress, unsigned int eeaddress, byte *buffer, int length ) {
Wire.beginTransmission(deviceaddress);
Wire.write((int)(eeaddress >> 8)); // MSB
Wire.write((int)(eeaddress & 0xFF)); // LSB
Wire.endTransmission();
Wire.requestFrom(deviceaddress,length);
int c = 0;
for ( c = 0; c < length; c++ )
if (Wire.available()) buffer[c] = Wire.read();
}
void setup()
{
char somedata[] = "this is data from the eeprom"; // data to write
Wire.begin(); // initialise the connection
Serial.begin(9600);
i2c_eeprom_write_page(0x50, 0, (byte *)somedata, sizeof(somedata)); // write to EEPROM
delay(10); //add a small delay
Serial.println("Memory written");
}
void loop()
{
int addr=0; //first address
byte b = i2c_eeprom_read_byte(0x50, 0); // access the first address from the memory
while (b!=0)
{
Serial.print((char)b); //print content to serial port
addr++; //increase address
b = i2c_eeprom_read_byte(0x50, addr); //access an address from the memory
}
Serial.println(" ");
delay(2000);
}
Results
More Documents
FAQ
For any questions, advice or cool ideas to share, please visit the DFRobot Forum.