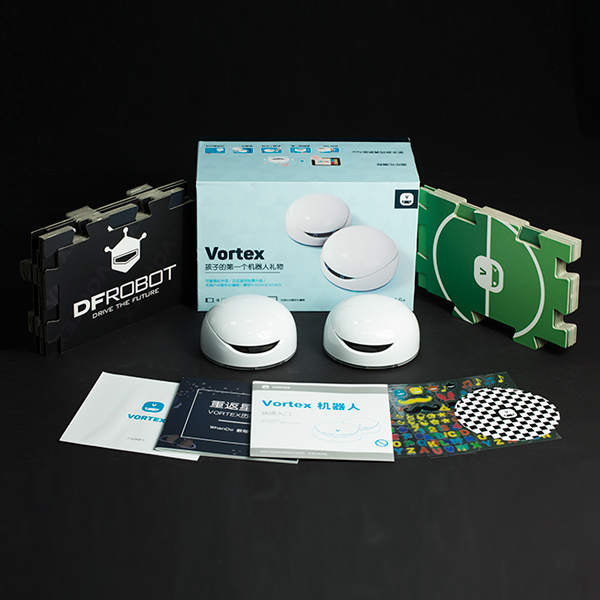
Introduction
Vortex is a revolutionary product for children. It is a smart and responsive robot that kids can play with and program. Using the Vortex and apps, kids can play different games, learn about robotics, and even create their own.
In this Tutorial, we we'll introduce vortex hardware features and teach how to program your vortex robot via Arduino IDE.
Specification
- MCU: ATmega328p (Bootloader: Arduino UNO)
- Operating voltage: 5V
- Power: 3.5~6V (4xAA Battery/USB)
- Size: Diameter: 120mm; Height: 64mm
- Net weight: 260g
- Support Bluetooth 4.0
- Programming Method: WhenDo/Scratch/Ardunio
Overview
- Feature:
- Bluetooth Communication
- Motor Control
- Line tracking
- Autonomous obstacle avoidance
- RGB LED Color Control
- MP3 Music Player
- Encoder
- Robot Eye
Programming Method
Vortex is a programmable robot based on Arduino open-source hardware. There are 2 kinds of programming methods: USB or Bluetooth wireless programming.
What You Need
- Software: Arduino IDE Click here to Download Arduino IDE from Arduino®
- Hardware:
- USB programming requires a micro USB cable only.
- Bluetooth wireless programming requires a USB BLE Link
USB Programming
- Unscrew the screw and open the USB programming port.
- Turn on Vortex power switch, and plug in the Micro usb cable, it will install the driver automatically if you have installed Arduino IDE. If not, you can find it in the driver file in Arduino IDE folder-->Drivers folder.
- There is a switch close to the USB port, make sure the trigger is close to the USB side. This is MP3 switch, we'll teach you how to add new song in the following chapter.
- Open your Arduino IDE, select "Arduino UNO" and right "COM port" in Arduino IDE, now you can enjoy coding.
Bluetooth Wireless Programming
BLE wireless programming could let you get rid of the mess USB cable, and make a free real-time debugging.
- Plug USB BLE Link to your PC (Compatible with Windows/MAC/Linux), and it will install the driver automatically if you have installed Arduino IDE. If not, you can find it in the driver file in Arduino IDE folder-->Drivers folder.
- Open your Arduino IDE, select "Arduino UNO" and right "COM port" in Arduino IDE, now you can enjoy coding.
Features
Bluetooth Communication
Vortex supports Bluetooth wireless communication, you can refer to the Bluno wiki page for the Bluetooth configuration and firmware upgrade.
Motor Control
Vortex implements PWM speed motor control, using 4 digital I/O pins:
- Left motor: D5(PWM); D9(Direction)
- Right motor: D6(PWM); D10(Direction)
Sample Code
int E1 = 5;
int M1 = 9;
int E2 = 6;
int M2 = 10;
void setup()
{
pinMode(M1, OUTPUT);
pinMode(M2, OUTPUT);
}
void loop()
{
int value;
for(value = 0 ; value <= 255; value+=5) //foreward
{
digitalWrite(M1,HIGH);
digitalWrite(M2, HIGH);
analogWrite(E1, value); //PWM Speed control
analogWrite(E2, value); //PWM Speed Control
delay(30);
}
for(value = 0 ; value <= 255; value+=5) //backward
{
digitalWrite(M1, LOW);
digitalWrite(M2, LOW);
analogWrite(E1, value); //PWM Speed control
analogWrite(E2, value); //PWM Speed Control
delay(30);
}
}
Line Tracking
Vortex has six Grayscale Sensors around the body. Achieving two-color detection. User can make a line tracking with these sensors.
| | |
| ------------------------------------------------------------------------------------------- | |
| | |
Num. | Pin |
---|---|
1 | A3 |
2 | A2 |
3 | A1 |
4 | A0 |
5 | A6 |
6 | A7 |
Driving Pins
The following code has separated motor part to make it more clear. It will read 6 gray value.
/***************************************************
Vortex V1.0 (Small robots like bread)
<https://www.dfrobot.com.cn/goods-1199.html>
***************************************************
This example show how to use Gray sensor on vortex.
Created 2016-2-3
By Andy zhou <Andy.zhou@dfrobot.com>
version:V1.0
****************************************************/
/***********Notice and Trouble shooting***************
1.Connection and Diagram can be found here
<http://wiki.dfrobot.com.cn/index.php?title=(SKU:ROB0116)_Vortex%E5%8F%AF%E7%BC%96%E7%A8%8B%E6%9C%BA%E5%99%A8%E4%BA%BA#.E6.A0.B7.E4.BE.8B.E4.BB.A3.E7.A0.81>
2.This code is tested on vortex V1.0.
****************************************************/
void setup(void){
Serial.begin(9600);
}
int analogBuf[6] = {'\0'};
void loop(void){
analogBuf[0] = analogRead(3);
analogBuf[1] = analogRead(2);
analogBuf[2] = analogRead(1);
analogBuf[3] = analogRead(0);
analogBuf[4] = analogRead(6);
analogBuf[5] = analogRead(7);
for(int i=0;i<6;i++){
Serial.print(i+1);
Serial.print(": ");
Serial.print(analogBuf[i]);
Serial.print(" ");
}
Serial.println();
delay(500);
}
Test Method You can leave a black strip in front of the Vortex, moving vortex cross the strip and checking the gray value one by one.
image:Vortex_test_method_1.jpg|Leave a black strip image:Vortex_test_method_2.png|All sensors are on the white paper image:Vortex_test_method_3.png|The first one is on the Black strip and the rest are on the white paper
Autonomous Obstacle Avoidance
Vortex is using 2 pairs of Infrared transmitter and receiver as its obstacle avoidance sensor. The transmitter will send 20 pulse at 38KHz frequency. Since the obstacle will reflect infrared light, we can judge the distance via the feedback pulse.
image:Vortex_Obstacle_Avoidance_1.png|Infrared Receiver image:Vortex_Obstacle_Avoidance_2.png|Infrared Transmitter
Driving Pins
- IR receiver pin: 7
- Left IR transmitter pin 8
- Right IR transmitter pin 12
The following code has separated motor part to make it more clear. It will detect whether there is something left or right
/***************************************************
Vortex V1.0 (Small robots like bread)
<https://www.dfrobot.com.cn/goods-1199.html>
***************************************************
This example show how to use Infrared sensor to avoid obstacles.
Created 2016-2-3
By Andy zhou <Andy.zhou@dfrobot.com>
version:V1.0
****************************************************/
/***********Notice and Trouble shooting***************
1.Connection and Diagram can be found here
<http://wiki.dfrobot.com.cn/index.php?title=(SKU:ROB0116)_Vortex%E5%8F%AF%E7%BC%96%E7%A8%8B%E6%9C%BA%E5%99%A8%E4%BA%BA#.E6.A0.B7.E4.BE.8B.E4.BB.A3.E7.A0.81>
2.This code is tested on vortex V1.0.
****************************************************/
#define IR_IN 7//IR receiver pin
#define L_IR 8 //left ir transmitter pin
#define R_IR 12 //right ir transmitter pin
int count;
void leftSend38KHZ(void){//left ir transmitter sends 38kHZ pulse
int i;
for(i=0;i<24;i++){
digitalWrite(L_IR,LOW);
delayMicroseconds(8);
digitalWrite(L_IR,HIGH);
delayMicroseconds(8);
}
}
void rightSend38KHZ(void){//right ir transmitter sends 38kHZ pulse
int i;
for(i=0;i<24;i++){
digitalWrite(R_IR,LOW);
delayMicroseconds(8);
digitalWrite(R_IR,HIGH);
delayMicroseconds(8);
}
}
void pcint0Init(void){//init the interrupt
PCICR |= 1 << PCIE2;
PCMSK2 |= 1 << PCINT23;
}
ISR(PCINT2_vect){//motor encoder interrupt
count++;
}
void obstacleAvoidance(void){
char i;
count=0;
for(i=0;i<20;i++){ //left transmitter sends 20 pulses
leftSend38KHZ();
delayMicroseconds(600);
}
if(count>20){//if recieved a lot pulse , it means there's a obstacle
Serial.println("Left");
delay(100);
}
count=0;
for(i=0;i<20;i++){//right transmitter sends 20 pulses
rightSend38KHZ();
delayMicroseconds(600);
}
if(count>20){//if recieved a lot pulse , it means there's a obstacle
Serial.println("Right");
delay(100);
}
}
void setup(void){
pinMode(L_IR,OUTPUT);//init the left transmitter pin
pinMode(R_IR,OUTPUT);//init the right transmitter pin
pinMode(IR_IN,INPUT);//init the ir receiver pin
Serial.begin(9600);
pcint0Init();
sei(); //enable the interrupt
}
void loop(void){
obstacleAvoidance();
}
After you upload the sample code, you can open the serial port to check the result. Leave your hand in front of the vortex, it will feedback your hand position.
RGB LED Color Control
Vortex has 6+6 RGB LEDs on the board, these LEDs integrates driving IC, you can control them via single signal.
Driving Pins
- RGB LEDs driving Pin: D13
[Link: Download Vortex Library] About Library installation
/***************************************************
Vortex V1.0 (Small robots like bread)
***************************************************
This example show how to use RGB-leds.
Created 2016-2-3
By Andy zhou <Andy.zhou@dfrobot.com>
version:V1.0
****************************************************/
/***********Notice and Trouble shooting***************
1.This code is tested on vortex V1.0.
****************************************************/
// Use if you want to force the software SPI subsystem to be used for some reason (generally, you don't)
// #define FORCE_SOFTWARE_SPI
// Use if you want to force non-accelerated pin access (hint: you really don't, it breaks lots of things)
// #define FORCE_SOFTWARE_SPI
// #define FORCE_SOFTWARE_PINS
#include "FastLED.h"
///////////////////////////////////////////////////////////////////////////////////////////
//
// Move a white dot along the strip of leds. This program simply shows how to configure the leds,
// and then how to turn a single pixel white and then off, moving down the line of pixels.
//
// How many leds are in the strip?
#define NUM_LEDS 12
// Data pin that led data will be written out over
#define DATA_PIN 13
// Clock pin only needed for SPI based chipsets when not using hardware SPI
//#define CLOCK_PIN 8
// This is an array of leds. One item for each led in your strip.
CRGB leds[NUM_LEDS];
// This function sets up the ledsand tells the controller about them
void setup() {
// sanity check delay - allows reprogramming if accidently blowing power w/leds
delay(2000);
// Uncomment one of the following lines for your leds arrangement.
// FastLED.addLeds<TM1803, DATA_PIN, RGB>(leds, NUM_LEDS);
// FastLED.addLeds<TM1804, DATA_PIN, RGB>(leds, NUM_LEDS);
// FastLED.addLeds<TM1809, DATA_PIN, RGB>(leds, NUM_LEDS);
FastLED.addLeds<WS2811, DATA_PIN, RGB>(leds, NUM_LEDS);
// FastLED.addLeds<WS2812, DATA_PIN, RGB>(leds, NUM_LEDS);
// FastLED.addLeds<WS2812B, DATA_PIN, RGB>(leds, NUM_LEDS);
// FastLED.addLeds<NEOPIXEL, DATA_PIN>(leds, NUM_LEDS);
// FastLED.addLeds<WS2811_400, DATA_PIN, RGB>(leds, NUM_LEDS);
// FastLED.addLeds<GW6205, DATA_PIN, RGB>(leds, NUM_LEDS);
// FastLED.addLeds<GW6205_400, DATA_PIN, RGB>(leds, NUM_LEDS);
// FastLED.addLeds<UCS1903, DATA_PIN, RGB>(leds, NUM_LEDS);
// FastLED.addLeds<UCS1903B, DATA_PIN, RGB>(leds, NUM_LEDS);
// FastLED.addLeds<WS2801, RGB>(leds, NUM_LEDS);
// FastLED.addLeds<SM16716, RGB>(leds, NUM_LEDS);
// FastLED.addLeds<LPD8806, RGB>(leds, NUM_LEDS);
// FastLED.addLeds<P9813, RGB>(leds, NUM_LEDS);
// FastLED.addLeds<WS2801, DATA_PIN, CLOCK_PIN, RGB>(leds, NUM_LEDS);
// FastLED.addLeds<SM16716, DATA_PIN, CLOCK_PIN, RGB>(leds, NUM_LEDS);
// FastLED.addLeds<LPD8806, DATA_PIN, CLOCK_PIN, RGB>(leds, NUM_LEDS);
}
// This function runs over and over, and is where you do the magic to light
// your leds.
void loop() {
// Move a single white led
for(int whiteLed = 0; whiteLed < NUM_LEDS; whiteLed = whiteLed + 1) {
// Turn our current led on to white, then show the leds
leds[whiteLed] = CRGB::White;
// Show the leds (only one of which is set to white, from above)
FastLED.show();
// Wait a little bit
delay(100);
// Turn our current led back to black for the next loop around
leds[whiteLed] = CRGB::Black;
}
}
MP3 Music Player
Do you still remember the MP3 Switch in the USB programming section? There is a switch close to the USB port, make sure the trigger is close to the USB side. This is MP3 switch, we'll teach you how to add new song in the following chapter.
Now here we go, we'll tell you how to replace vortex system voice with your own one.
- Turn the switch trigger to the opposite side (away from the USB port).
- Connect Vortex to your computer via USB port. and it will appear a new flash disk.
- Rename your voice or your song file, and put them in the disk.
NOTE: The MP3 chip recognizes the file by the modified time, not its name, so you will need to rank the file again! Just cut all file outside, and move them in the disk in order. |
/***************************************************
Vortex V1.0 (Small robots like bread)
<https://www.dfrobot.com.cn/goods-1199.html>
***************************************************
This example show how to device mp3 player.
Created 2016-2-3
By Andy zhou <Andy.zhou@dfrobot.com>
version:V1.0
****************************************************/
/***********Notice and Trouble shooting***************
1.Connection and Diagram can be found here
<http://wiki.dfrobot.com.cn/index.php?title=(SKU:ROB0116)_Vortex%E5%8F%AF%E7%BC%96%E7%A8%8B%E6%9C%BA%E5%99%A8%E4%BA%BA#.E6.A0.B7.E4.BE.8B.E4.BB.A3.E7.A0.81>
2.This code is tested on vortex V1.0.
****************************************************/
#include <SoftwareSerial.h>
#include <VortexMp3.h>
#define MP3_VOLUME 0x10
void init(){
mp3.Init();
mp3.setVolume(MP3_VOLUME);
}
void setup() {
// put your setup code here, to run once:
init();
}
static int musicState = 1;
void loop(){
// put your main code here, to run repeatedly:
mp3.player(musicState);
musicState++;
if (musicState>=20){
musicState = 1;
}
delay(1000);
}
Encoder
Vortex is using optical-electricity encoder.
Driving Pins
- Left Encoder: Interrupt 0 (D2)
- Right Encoder: Interrupt 1 (D3)
Sample Code
/***************************************************
Vortex V1.0 (Small robots like bread)
<https://www.dfrobot.com.cn/goods-1199.html>
***************************************************
This example show how to use encoder sensor.
Created 2016-2-3
By Andy zhou <Andy.zhou@dfrobot.com>
version:V1.0
****************************************************/
/***********Notice and Trouble shooting***************
1.Connection and Diagram can be found here
<http://wiki.dfrobot.com.cn/index.php?title=(SKU:ROB0116)_Vortex%E5%8F%AF%E7%BC%96%E7%A8%8B%E6%9C%BA%E5%99%A8%E4%BA%BA#.E6.A0.B7.E4.BE.8B.E4.BB.A3.E7.A0.81>
2.This code is tested on vortex V1.0.
****************************************************/
#define pinInputLeft 0
#define pinInputRight 1
long leftPul,rightPul;
void leftCallBack(){
leftPul++;
}
void rightCallBack(){
rightPul++;
}
void initDdevice(){
pinMode(5,OUTPUT);
pinMode(6,OUTPUT);
pinMode(9,OUTPUT);
pinMode(10,OUTPUT);
attachInterrupt(pinInputLeft,leftCallBack,CHANGE);
attachInterrupt(pinInputRight,rightCallBack,CHANGE);
sei();
}
void motorDebug(){
digitalWrite(5,HIGH);
digitalWrite(6,HIGH);
digitalWrite(9,HIGH);
digitalWrite(10,HIGH);
}
void printPul(){
Serial.print(leftPul);
Serial.print(" ");
Serial.println(rightPul);
leftPul = 0;
rightPul = 0;
}
void setup() {
initDdevice();
Serial.begin(9600);
motorDebug();
}
void loop() {
printPul();
delay(500);
}
Result
Robot Eye
Vortex uses two 5x5 leds matrix as its eye, IIC interface.
/***************************************************
Vortex V1.0 (Small robots like bread)
<https://www.dfrobot.com.cn/goods-1199.html>
***************************************************
This example show how to use Gray sensor on vortex.
Created 2016-2-3
By Andy zhou <Andy.zhou@dfrobot.com>
version:V1.0
****************************************************/
/***********Notice and Trouble shooting***************
1.Connection and Diagram can be found here
<http://wiki.dfrobot.com.cn/index.php?title=(SKU:ROB0116)_Vortex%E5%8F%AF%E7%BC%96%E7%A8%8B%E6%9C%BA%E5%99%A8%E4%BA%BA#.E6.A0.B7.E4.BE.8B.E4.BB.A3.E7.A0.81>
2.This code is tested on vortex V1.0.
****************************************************/
#include <Wire.h>
#define I2C_LED_ADDRESS 0b1100000
#define I2C_WRITE 0x00
uint8_t serial=0;
void setup(){
Wire.begin(); // join i2c bus (address optional for master)
}
void loop(){
Wire.beginTransmission(I2C_LED_ADDRESS << 1 | I2C_WRITE); // transmit to device #4
Wire.write(0x01);
Wire.write(serial);
/*Wire.write(0x55);
Wire.write(0xAA);
Wire.write(0x07); //color set
//1 2 3 4 5 25 24 23 22 21
//6 7 8 9 10 20 19 18 17 16
//11 12 13 14 15 15 14 13 12 11
//16 17 18 19 20 10 9 8 7 6
//21 22 23 24 25 5 4 3 2 1
Wire.write(0x07);
Wire.write(0x18);
Wire.write(0x00);
Wire.write(0x04);
Wire.write(0x00);
Wire.write(0x07);
Wire.write(0x18);
Wire.write(0x00);
Wire.write(0x04);
Wire.write(0x00);
Wire.endTransmission(); // stop transmitting
serial++;
if(serial==35) serial=0;
delay(500);
}