Introduction
The face recognition module is mainly composed of a professional-grade video application processor and a binocular 3D infrared camera module. It has a self-developed embedded system 3D depth restoration algorithm, 3D face recognition algorithm and multi-modal liveness prevention algorithm, which can effectively protect user information and unlocking security. The face recognition module can store 100 faces, with a recognition speed of 1s. At the same time, it uses a multi-modal liveness anti-counterfeiting algorithm to effectively block attacks from photos, videos, and various head models and dummies.
The face recognition module uses a Gravity interface, supports UART and I2C communication methods, and is compatible with Arduino Uno, Arduino Leonardo, Arduino MEGA, FireBeetle series controllers, Raspberry Pi, ESP32 and other main controllers. It can be used in smart homes, smart door locks, smart access control, attendance machines, 3D face payment systems, smart device unlocking and other scenarios.
Features
- Recognition speed 1s
- High-performance algorithm chip
- Supports storage of 100 faces
- Liveness detection, resistance to various attacks
- Binocular 3D infrared camera, available in dark environments
- Adopts Gravity interface, supports UART and I2C communication methods
Specifications:
- Working voltage: 5V
- Maximum working current: ≤400mA
- Power: ≤2W
- Communication method: UART&I2C
- Identification angle:20° up, down, left and right
- Recognition time: 1s
- Number of faces stored: 100
- Recognition distance: 30~110cm
- Recognition height: 1.35~2.2m (20° inclination)
- Recognition rate: 98.85%
- False recognition rate: 0.0001%
- False acceptance rate LPFAR: ≤1%
- False rejection rate LPFRR: ≤1%
- Working temperature: -20°C~60°C
- Working humidity: 10~90%RH
Board Overview
Name | Function Description |
---|---|
D/T | I2C data signal/UART TX data line |
C/R | I2C clock signal/UART RX data line |
GND | GND |
5V | Power input positive pole |
Dimensional Drawing
Tutorial
Requirements
- Hardware
- DFRduino UNO R3 (or similar) x 1
- Gravity: 3D Face Recognition Module - UART&I2C x1
- Software
UART usage
UART Wiring diagram
Note: Due to the high power consumption of the face recognition module, the working current is ≤400mA, and the maximum is about 2W. The VCC power supply of the Arduino digital interface cannot meet the demand. The VCC power supply of the analog port and IC2 interface can be used.
Switch the communication mode dial switch to the UART end
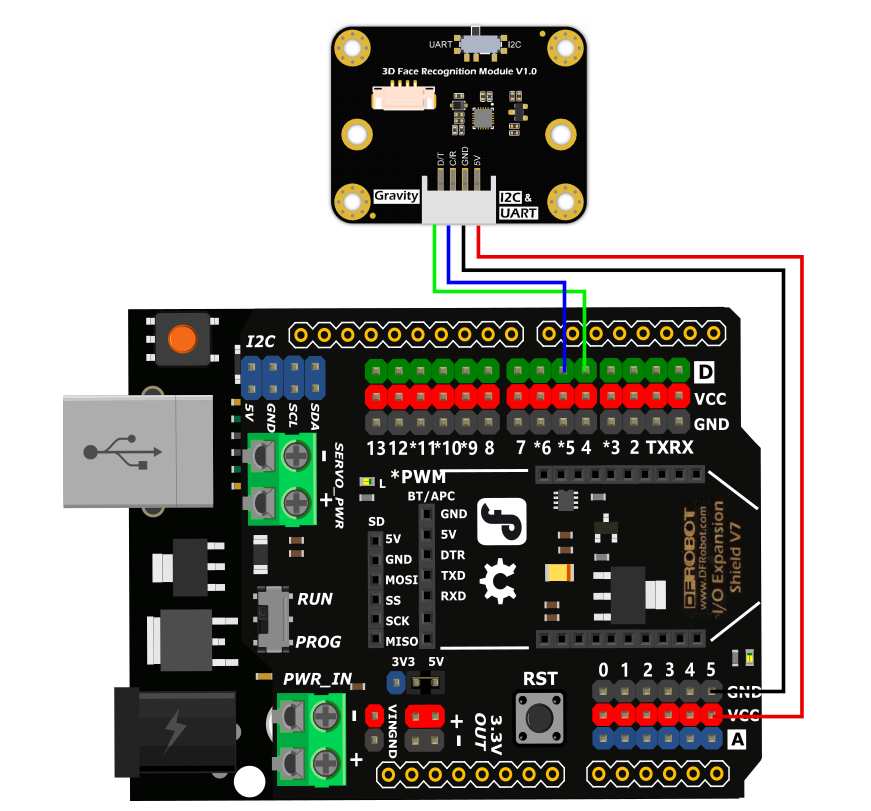
Example code 1 - Register one-dimensional face
Burn the code, the red LED lights up, aim the face module at the face to register the face, and only register one face at a time. If you need to register multiple faces, you can press the RST button to register other faces again.
/*!
* @file faceRecognition.ino
* @brief face recognition demo
* @copyright Copyright (c) 2010 DFRobot Co.Ltd (http://www.dfrobot.com)
* @license The MIT License (MIT)
* @author ZhixinLiu(zhixin.liu@dfrobot.com)
* @version V1.0
* @date 2023-12-07
* @url https://github.com/DFRobot/DFRobot_3DFace
*/
#include "DFRobot_3DFace.h"
//#define I2C_COMMUNICATION //use I2C for communication, but use the serial port for communication if the line of codes were masked
#ifdef I2C_COMMUNICATION
DFRobot_3DFace_I2C face(&Wire ,DEVICE_ADDR);
#else
/* ---------------------------------------------------------------------------------------------------------------------
* board | MCU | Leonardo/Mega2560/M0 | UNO | ESP8266 | ESP32 | microbit | m0 |
* VCC | 3.3V/5V | VCC | VCC | VCC | VCC | X | vcc |
* GND | GND | GND | GND | GND | GND | X | gnd |
* RX | TX | Serial1 TX1 | 5 | 5/D6 | D2 | X | tx1 |
* TX | RX | Serial1 RX1 | 4 | 4/D7 | D3 | X | rx1 |
* ----------------------------------------------------------------------------------------------------------------------*/
/* Baud rate cannot be changed */
#if defined(ARDUINO_AVR_UNO) || defined(ESP8266)
SoftwareSerial mySerial(4, 5);
DFRobot_3DFace_UART face(&mySerial ,57600);
#elif defined(ESP32)
DFRobot_3DFace_UART face(&Serial1 ,57600 ,/*rx*/D2 ,/*tx*/D3);
#else
DFRobot_3DFace_UART face(&Serial1 ,57600);
#endif
#endif
void setup()
{
Serial.begin(115200);
while(!Serial);
randomSeed(analogRead(A0));
while(!face.begin()){
Serial.println("NO Deivces !");
delay(1000);
} Serial.println("Device connected!");
sUserData_t data = face.getFaceMessage();
if(data.result == true){
Serial.print("user number = ");
Serial.println(data.user_count);
}
Serial.println("face resgistering !");
char rName[40] = {0};
uint16_t randNumber = random(analogRead(A0));
sprintf(rName, "rName%d", randNumber);
sFaceReg_t faceReg = face.faceRegistration(rName);
if(faceReg.result){
Serial.println("face resgistering success!");
Serial.print("regiseter user name = ");
Serial.println(rName);
Serial.print("regiseter user id = ");
Serial.println(faceReg.userID);
Serial.print("register direction = ");
Serial.println(faceReg.direction);
Serial.println();
}else{
Serial.print("face resgistering faild cause = ");
Serial.println(face.anaysisCode((eResponseCode_t)faceReg.errorCode));
}
}
void loop()
{
delay(1000);
}
Result
Register one-dimensional face once, and print the corresponding data on the serial port
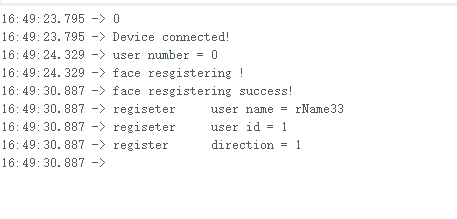
Example code 2 - Register five-dimensional face
After burning the code, follow the steps of serial port print data to register five-dimensional face, which are straight, look up, look down, look left, and look right. Only one face is registered each time. If you need to register multiple faces, you can press the RST button to register other faces again.
/*!
* @file faceRecognition.ino
* @brief face recognition demo
* @copyright Copyright (c) 2010 DFRobot Co.Ltd (http://www.dfrobot.com)
* @license The MIT License (MIT)
* @author ZhixinLiu(zhixin.liu@dfrobot.com)
* @version V1.0
* @date 2023-12-07
* @url https://github.com/DFRobot/DFRobot_3DFace
*/
#include "DFRobot_3DFace.h"
// #define I2C_COMMUNICATION //use I2C for communication, but use the serial port for communication if the line of codes were masked
#ifdef I2C_COMMUNICATION
DFRobot_3DFace_I2C face(&Wire ,DEVICE_ADDR);
#else
/* ---------------------------------------------------------------------------------------------------------------------
* board | MCU | Leonardo/Mega2560/M0 | UNO | ESP8266 | ESP32 | microbit | m0 |
* VCC | 3.3V/5V | VCC | VCC | VCC | VCC | X | vcc |
* GND | GND | GND | GND | GND | GND | X | gnd |
* RX | TX | Serial1 TX1 | 5 | 5/D6 | D2 | X | tx1 |
* TX | RX | Serial1 RX1 | 4 | 4/D7 | D3 | X | rx1 |
* ----------------------------------------------------------------------------------------------------------------------*/
/* Baud rate cannot be changed */
#if defined(ARDUINO_AVR_UNO) || defined(ESP8266)
SoftwareSerial mySerial(4, 5);
DFRobot_3DFace_UART face(&mySerial ,57600);
#elif defined(ESP32)
DFRobot_3DFace_UART face(&Serial1 ,57600 ,/*rx*/D2 ,/*tx*/D3);
#else
DFRobot_3DFace_UART face(&Serial1 ,57600);
#endif
#endif
void setup()
{
Serial.begin(115200);
while(!Serial);
randomSeed(analogRead(A0));
while(!face.begin()){
Serial.println("NO Deivces !");
delay(1000);
} Serial.println("Device connected!");
sUserData_t data = face.getFaceMessage();
if(data.result == true){
Serial.print("user number = ");
Serial.println(data.user_count);
}
char rName[40] = {0};
Serial.println("face resgistering !");
Serial.println("Please look straight into the camera.");
sFaceReg_t faceReg = face.directRegistration();
if(faceReg.result){
Serial.print("register direction = ");
Serial.println(faceReg.direction);
Serial.println("Direct view record success");
Serial.println("Please look up.");
faceReg = face.lookUpRegistration();
}
if(faceReg.result){
Serial.print("register direction = ");
Serial.println(faceReg.direction);
Serial.println("look up record success");
Serial.println("Please look down.");
faceReg = face.lookDownRegistration();
}
if(faceReg.result){
Serial.print("register direction = ");
Serial.println(faceReg.direction);
Serial.println("look down record success");
Serial.println("Please look to the left.");
faceReg = face.turnLeftRegistration();
}
if(faceReg.result){
Serial.print("register direction = ");
Serial.println(faceReg.direction);
Serial.println("look left record success");
Serial.println("Please look to the right.");
uint16_t randNumber = random(analogRead(A0));
sprintf(rName, "fiveName%d", randNumber);
faceReg = face.turnRightRegistration(rName);
}
if(faceReg.result){
Serial.println("five face resgistering success!");
Serial.print("regiseter user name = ");
Serial.println(rName);
Serial.print("regiseter user id = ");
Serial.println(faceReg.userID);
}else{
Serial.print("five face resgistering faild cause = ");
Serial.println(face.anaysisCode((eResponseCode_t)faceReg.errorCode));
}
}
void loop()
{
delay(1000);
}
Result
Register a five-dimensional face according to the serial port printing data steps. Each step will provide feedback on whether the recognition is successful.
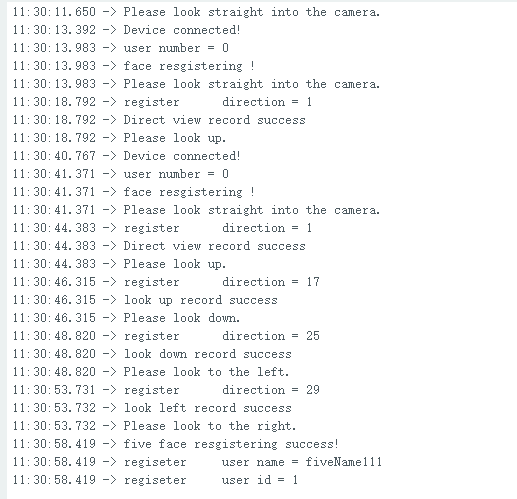
Example code 3 - Recognize face
Burn the code, the red LED lights up, and aim the face module at the face. At this time, the face will be recognized in a loop.
/*!
* @file faceMatching.ino
* @brief face matching demo
* @copyright Copyright (c) 2010 DFRobot Co.Ltd (http://www.dfrobot.com)
* @license The MIT License (MIT)
* @author ZhixinLiu(zhixin.liu@dfrobot.com)
* @version V1.0
* @date 2024-04-25
* @url https://github.com/DFRobot/DFRobot_3DFace
*/
#include "DFRobot_3DFace.h"
//#define I2C_COMMUNICATION //use I2C for communication, but use the serial port for communication if the line of codes were masked
#ifdef I2C_COMMUNICATION
DFRobot_3DFace_I2C face(&Wire ,DEVICE_ADDR);
#else
/* ---------------------------------------------------------------------------------------------------------------------
* board | MCU | Leonardo/Mega2560/M0 | UNO | ESP8266 | ESP32 | microbit | m0 |
* VCC | 3.3V/5V | VCC | VCC | VCC | VCC | X | vcc |
* GND | GND | GND | GND | GND | GND | X | gnd |
* RX | TX | Serial1 TX1 | 5 | 5/D6 | D2 | X | tx1 |
* TX | RX | Serial1 RX1 | 4 | 4/D7 | D3 | X | rx1 |
* ----------------------------------------------------------------------------------------------------------------------*/
/* Baud rate cannot be changed */
#if defined(ARDUINO_AVR_UNO) || defined(ESP8266)
SoftwareSerial mySerial(4, 5);
DFRobot_3DFace_UART face(&mySerial ,57600);
#elif defined(ESP32)
DFRobot_3DFace_UART face(&Serial1 ,57600 ,/*rx*/D2 ,/*tx*/D3);
#else
DFRobot_3DFace_UART face(&Serial1 ,57600);
#endif
#endif
void setup()
{
Serial.begin(115200);
while(!Serial);
while(!face.begin()){
Serial.println("NO Deivces !");
delay(1000);
} Serial.println("Device connected!");
sUserData_t data = face.getFaceMessage();
if(data.result == true){
Serial.print("user number = ");
Serial.println(data.user_count);
}
}
void loop()
{
Serial.println("face matching ..............");
sFaceMatching_t matching = face.faceMatching();
if(matching.result){
Serial.println("face matching success!");
Serial.print("matching user id = ");
Serial.println(matching.userID);
Serial.print("matching name = ");
Serial.println((char*)matching.name);
Serial.println();
}else{
Serial.print("face matching faild cause is ");
Serial.println(face.anaysisCode((eResponseCode_t)matching.errorCode));
}
delay(1000);
}
Result
The detected faces are identified in a loop, and the serial port prints the corresponding data.
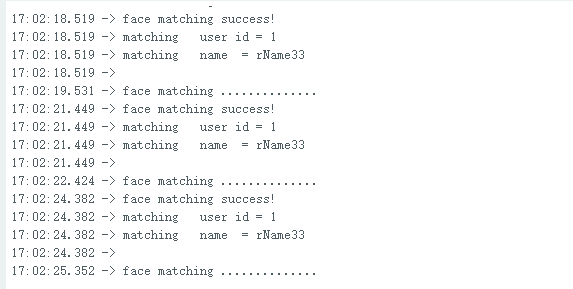
Example code 4 - Delete face
After burning the code, the specified ID face or all faces will be deleted. Changing the ID can delete other specified faces.
/*!
* @file faceDelete.ino
* @brief face delete demo
* @copyright Copyright (c) 2010 DFRobot Co.Ltd (http://www.dfrobot.com)
* @license The MIT License (MIT)
* @author ZhixinLiu(zhixin.liu@dfrobot.com)
* @version V1.0
* @date 2024-04-25
* @url https://github.com/DFRobot/DFRobot_3DFace
*/
#include "DFRobot_3DFace.h"
//#define I2C_COMMUNICATION //use I2C for communication, but use the serial port for communication if the line of codes were masked
#ifdef I2C_COMMUNICATION
DFRobot_3DFace_I2C face(&Wire ,DEVICE_ADDR);
#else
/* ---------------------------------------------------------------------------------------------------------------------
* board | MCU | Leonardo/Mega2560/M0 | UNO | ESP8266 | ESP32 | microbit | m0 |
* VCC | 3.3V/5V | VCC | VCC | VCC | VCC | X | vcc |
* GND | GND | GND | GND | GND | GND | X | gnd |
* RX | TX | Serial1 TX1 | 5 | 5/D6 | D2 | X | tx1 |
* TX | RX | Serial1 RX1 | 4 | 4/D7 | D3 | X | rx1 |
* ----------------------------------------------------------------------------------------------------------------------*/
/* Baud rate cannot be changed */
#if defined(ARDUINO_AVR_UNO) || defined(ESP8266)
SoftwareSerial mySerial(4, 5);
DFRobot_3DFace_UART face(&mySerial ,57600);
#elif defined(ESP32)
DFRobot_3DFace_UART face(&Serial1 ,57600 ,/*rx*/D2 ,/*tx*/D3);
#else
DFRobot_3DFace_UART face(&Serial1 ,57600);
#endif
#endif
void setup()
{
Serial.begin(115200);
while(!face.begin()){
Serial.println("NO Deivces !");
delay(1000);
} Serial.println("Device connected!");
sUserData_t data = face.getFaceMessage();
if(data.result == true){
Serial.print("user number = ");
Serial.println(data.user_count);
}
/*
if(face.delAllFaceID()){
Serial.println("delete all face success!");
}
*/
uint8_t number = 1;
if(face.delFaceID(number)){
Serial.print("success delete face id = ");
Serial.println(number);
}else{
Serial.print("faild delete face id = ");
Serial.println(number);
}
}
void loop()
{
sUserData_t data = face.getFaceMessage();
if(data.result == true){
Serial.print("user number = ");
Serial.println(data.user_count);
}
delay(1000);
}
Result
Delete the specified ID face or all faces, and the serial port prints the corresponding data.
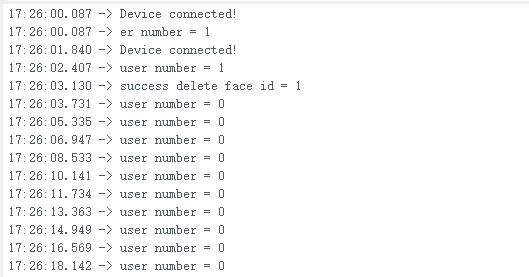
I2C usage
I2C Wiring diagram
Turn the dial switch for switching communication mode to the I2C end
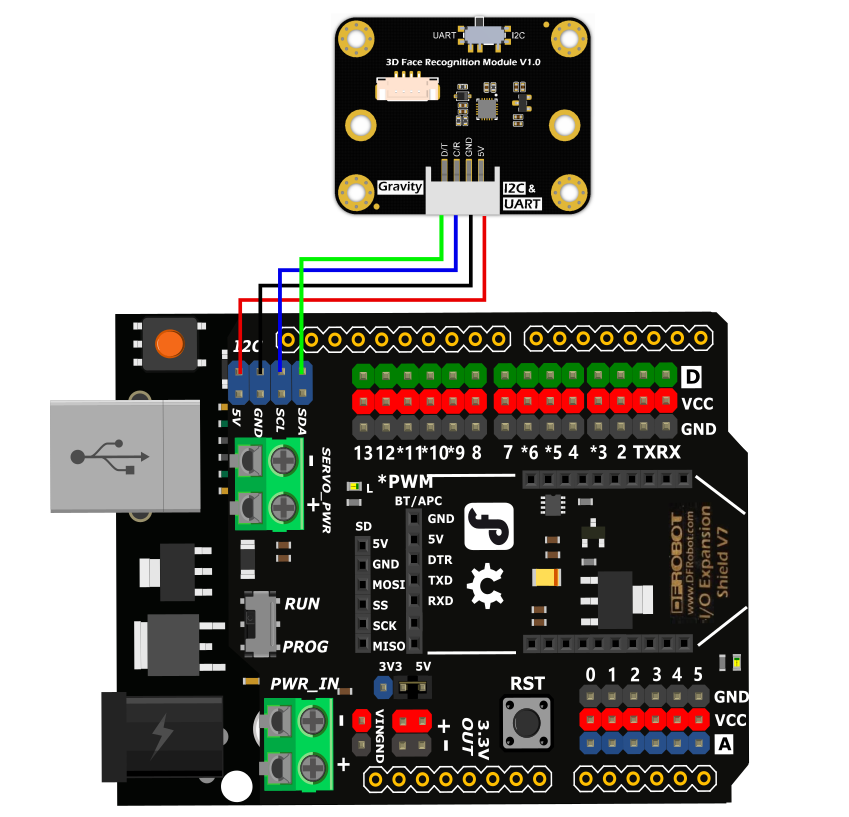
Example code 1 - Register face
Burn the code, the red LED lights up, aim the face module at the face to register the face, and only register one face at a time. If you need to register multiple faces, you can press the RST button to register other faces again.
/*!
* @file faceRecognition.ino
* @brief face recognition demo
* @copyright Copyright (c) 2010 DFRobot Co.Ltd (http://www.dfrobot.com)
* @license The MIT License (MIT)
* @author ZhixinLiu(zhixin.liu@dfrobot.com)
* @version V1.0
* @date 2023-12-07
* @url https://github.com/DFRobot/DFRobot_3DFace
*/
#include "DFRobot_3DFace.h"
#define I2C_COMMUNICATION //use I2C for communication, but use the serial port for communication if the line of codes were masked
#ifdef I2C_COMMUNICATION
DFRobot_3DFace_I2C face(&Wire ,DEVICE_ADDR);
#else
/* ---------------------------------------------------------------------------------------------------------------------
* board | MCU | Leonardo/Mega2560/M0 | UNO | ESP8266 | ESP32 | microbit | m0 |
* VCC | 3.3V/5V | VCC | VCC | VCC | VCC | X | vcc |
* GND | GND | GND | GND | GND | GND | X | gnd |
* RX | TX | Serial1 TX1 | 5 | 5/D6 | D2 | X | tx1 |
* TX | RX | Serial1 RX1 | 4 | 4/D7 | D3 | X | rx1 |
* ----------------------------------------------------------------------------------------------------------------------*/
/* Baud rate cannot be changed */
#if defined(ARDUINO_AVR_UNO) || defined(ESP8266)
SoftwareSerial mySerial(4, 5);
DFRobot_3DFace_UART face(&mySerial ,57600);
#elif defined(ESP32)
DFRobot_3DFace_UART face(&Serial1 ,57600 ,/*rx*/D2 ,/*tx*/D3);
#else
DFRobot_3DFace_UART face(&Serial1 ,57600);
#endif
#endif
void setup()
{
Serial.begin(115200);
while(!Serial);
randomSeed(analogRead(A0));
while(!face.begin()){
Serial.println("NO Deivces !");
delay(1000);
} Serial.println("Device connected!");
sUserData_t data = face.getFaceMessage();
if(data.result == true){
Serial.print("user number = ");
Serial.println(data.user_count);
}
Serial.println("face resgistering !");
char rName[40] = {0};
uint16_t randNumber = random(analogRead(A0));
sprintf(rName, "rName%d", randNumber);
sFaceReg_t faceReg = face.faceRegistration(rName);
if(faceReg.result){
Serial.println("face resgistering success!");
Serial.print("regiseter user name = ");
Serial.println(rName);
Serial.print("regiseter user id = ");
Serial.println(faceReg.userID);
Serial.print("register direction = ");
Serial.println(faceReg.direction);
Serial.println();
}else{
Serial.print("face resgistering faild cause = ");
Serial.println(face.anaysisCode((eResponseCode_t)faceReg.errorCode));
}
}
void loop()
{
delay(1000);
}
Result
Register a face once, and the serial port prints the corresponding data
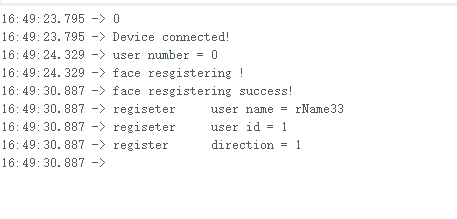
Example code 2 - Register five-dimensional face
After burning the code, follow the steps of serial port print data to register five-dimensional face, which are straight, look up, look down, look left, and look right. Only one face is registered each time. If you need to register multiple faces, you can press the RST button to register other faces again.
/*!
* @file faceRecognition.ino
* @brief face recognition demo
* @copyright Copyright (c) 2010 DFRobot Co.Ltd (http://www.dfrobot.com)
* @license The MIT License (MIT)
* @author ZhixinLiu(zhixin.liu@dfrobot.com)
* @version V1.0
* @date 2023-12-07
* @url https://github.com/DFRobot/DFRobot_3DFace
*/
#include "DFRobot_3DFace.h"
#define I2C_COMMUNICATION //use I2C for communication, but use the serial port for communication if the line of codes were masked
#ifdef I2C_COMMUNICATION
DFRobot_3DFace_I2C face(&Wire ,DEVICE_ADDR);
#else
/* ---------------------------------------------------------------------------------------------------------------------
* board | MCU | Leonardo/Mega2560/M0 | UNO | ESP8266 | ESP32 | microbit | m0 |
* VCC | 3.3V/5V | VCC | VCC | VCC | VCC | X | vcc |
* GND | GND | GND | GND | GND | GND | X | gnd |
* RX | TX | Serial1 TX1 | 5 | 5/D6 | D2 | X | tx1 |
* TX | RX | Serial1 RX1 | 4 | 4/D7 | D3 | X | rx1 |
* ----------------------------------------------------------------------------------------------------------------------*/
/* Baud rate cannot be changed */
#if defined(ARDUINO_AVR_UNO) || defined(ESP8266)
SoftwareSerial mySerial(4, 5);
DFRobot_3DFace_UART face(&mySerial ,57600);
#elif defined(ESP32)
DFRobot_3DFace_UART face(&Serial1 ,57600 ,/*rx*/D2 ,/*tx*/D3);
#else
DFRobot_3DFace_UART face(&Serial1 ,57600);
#endif
#endif
void setup()
{
Serial.begin(115200);
while(!Serial);
randomSeed(analogRead(A0));
while(!face.begin()){
Serial.println("NO Deivces !");
delay(1000);
} Serial.println("Device connected!");
sUserData_t data = face.getFaceMessage();
if(data.result == true){
Serial.print("user number = ");
Serial.println(data.user_count);
}
char rName[40] = {0};
Serial.println("face resgistering !");
Serial.println("Please look straight into the camera.");
sFaceReg_t faceReg = face.directRegistration();
if(faceReg.result){
Serial.print("register direction = ");
Serial.println(faceReg.direction);
Serial.println("Direct view record success");
Serial.println("Please look up.");
faceReg = face.lookUpRegistration();
}
if(faceReg.result){
Serial.print("register direction = ");
Serial.println(faceReg.direction);
Serial.println("look up record success");
Serial.println("Please look down.");
faceReg = face.lookDownRegistration();
}
if(faceReg.result){
Serial.print("register direction = ");
Serial.println(faceReg.direction);
Serial.println("look down record success");
Serial.println("Please look to the left.");
faceReg = face.turnLeftRegistration();
}
if(faceReg.result){
Serial.print("register direction = ");
Serial.println(faceReg.direction);
Serial.println("look left record success");
Serial.println("Please look to the right.");
uint16_t randNumber = random(analogRead(A0));
sprintf(rName, "fiveName%d", randNumber);
faceReg = face.turnRightRegistration(rName);
}
if(faceReg.result){
Serial.println("five face resgistering success!");
Serial.print("regiseter user name = ");
Serial.println(rName);
Serial.print("regiseter user id = ");
Serial.println(faceReg.userID);
}else{
Serial.print("five face resgistering faild cause = ");
Serial.println(face.anaysisCode((eResponseCode_t)faceReg.errorCode));
}
}
void loop()
{
delay(1000);
}
Result
Register a five-dimensional face according to the serial port printing data steps. Each step will provide feedback on whether the recognition is successful.
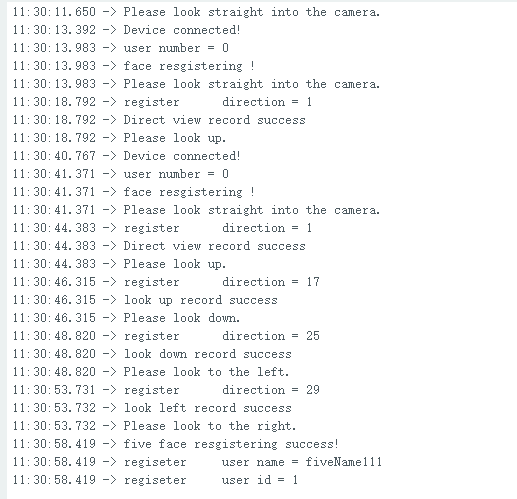
Example code 3 - Recognize face
Burn the code, the red LED lights up, and aim the face module at the face. At this time, the face will be recognized in a loop.
/*!
* @file faceMatching.ino
* @brief face matching demo
* @copyright Copyright (c) 2010 DFRobot Co.Ltd (http://www.dfrobot.com)
* @license The MIT License (MIT)
* @author ZhixinLiu(zhixin.liu@dfrobot.com)
* @version V1.0
* @date 2024-04-25
* @url https://github.com/DFRobot/DFRobot_3DFace
*/
#include "DFRobot_3DFace.h"
#define I2C_COMMUNICATION //use I2C for communication, but use the serial port for communication if the line of codes were masked
#ifdef I2C_COMMUNICATION
DFRobot_3DFace_I2C face(&Wire ,DEVICE_ADDR);
#else
/* ---------------------------------------------------------------------------------------------------------------------
* board | MCU | Leonardo/Mega2560/M0 | UNO | ESP8266 | ESP32 | microbit | m0 |
* VCC | 3.3V/5V | VCC | VCC | VCC | VCC | X | vcc |
* GND | GND | GND | GND | GND | GND | X | gnd |
* RX | TX | Serial1 TX1 | 5 | 5/D6 | D2 | X | tx1 |
* TX | RX | Serial1 RX1 | 4 | 4/D7 | D3 | X | rx1 |
* ----------------------------------------------------------------------------------------------------------------------*/
/* Baud rate cannot be changed */
#if defined(ARDUINO_AVR_UNO) || defined(ESP8266)
SoftwareSerial mySerial(4, 5);
DFRobot_3DFace_UART face(&mySerial ,57600);
#elif defined(ESP32)
DFRobot_3DFace_UART face(&Serial1 ,57600 ,/*rx*/D2 ,/*tx*/D3);
#else
DFRobot_3DFace_UART face(&Serial1 ,57600);
#endif
#endif
void setup()
{
Serial.begin(115200);
while(!Serial);
while(!face.begin()){
Serial.println("NO Deivces !");
delay(1000);
} Serial.println("Device connected!");
sUserData_t data = face.getFaceMessage();
if(data.result == true){
Serial.print("user number = ");
Serial.println(data.user_count);
}
}
void loop()
{
Serial.println("face matching ..............");
sFaceMatching_t matching = face.faceMatching();
if(matching.result){
Serial.println("face matching success!");
Serial.print("matching user id = ");
Serial.println(matching.userID);
Serial.print("matching name = ");
Serial.println((char*)matching.name);
Serial.println();
}else{
Serial.print("face matching faild cause is ");
Serial.println(face.anaysisCode((eResponseCode_t)matching.errorCode));
}
delay(1000);
}
Result
The detected faces are identified in a loop, and the serial port prints the corresponding data.
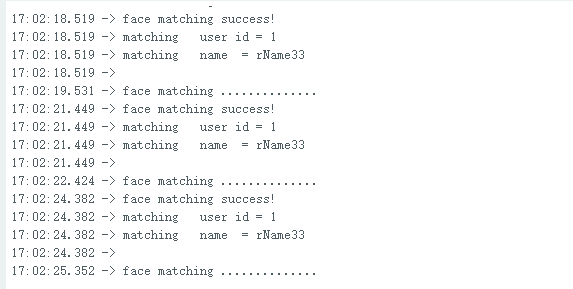
Example code 4 - Delete face
After burning the code, the specified ID face or all faces will be deleted. Changing the ID can delete other specified faces.
/*!
* @file faceDelete.ino
* @brief face delete demo
* @copyright Copyright (c) 2010 DFRobot Co.Ltd (http://www.dfrobot.com)
* @license The MIT License (MIT)
* @author ZhixinLiu(zhixin.liu@dfrobot.com)
* @version V1.0
* @date 2024-04-25
* @url https://github.com/DFRobot/DFRobot_3DFace
*/
#include "DFRobot_3DFace.h"
#define I2C_COMMUNICATION //use I2C for communication, but use the serial port for communication if the line of codes were masked
#ifdef I2C_COMMUNICATION
DFRobot_3DFace_I2C face(&Wire ,DEVICE_ADDR);
#else
/* ---------------------------------------------------------------------------------------------------------------------
* board | MCU | Leonardo/Mega2560/M0 | UNO | ESP8266 | ESP32 | microbit | m0 |
* VCC | 3.3V/5V | VCC | VCC | VCC | VCC | X | vcc |
* GND | GND | GND | GND | GND | GND | X | gnd |
* RX | TX | Serial1 TX1 | 5 | 5/D6 | D2 | X | tx1 |
* TX | RX | Serial1 RX1 | 4 | 4/D7 | D3 | X | rx1 |
* ----------------------------------------------------------------------------------------------------------------------*/
/* Baud rate cannot be changed */
#if defined(ARDUINO_AVR_UNO) || defined(ESP8266)
SoftwareSerial mySerial(4, 5);
DFRobot_3DFace_UART face(&mySerial ,57600);
#elif defined(ESP32)
DFRobot_3DFace_UART face(&Serial1 ,57600 ,/*rx*/D2 ,/*tx*/D3);
#else
DFRobot_3DFace_UART face(&Serial1 ,57600);
#endif
#endif
void setup()
{
Serial.begin(115200);
while(!face.begin()){
Serial.println("NO Deivces !");
delay(1000);
} Serial.println("Device connected!");
sUserData_t data = face.getFaceMessage();
if(data.result == true){
Serial.print("user number = ");
Serial.println(data.user_count);
}
/*
if(face.delAllFaceID()){
Serial.println("delete all face success!");
}
*/
uint8_t number = 1;
if(face.delFaceID(number)){
Serial.print("success delete face id = ");
Serial.println(number);
}else{
Serial.print("faild delete face id = ");
Serial.println(number);
}
}
void loop()
{
sUserData_t data = face.getFaceMessage();
if(data.result == true){
Serial.print("user number = ");
Serial.println(data.user_count);
}
delay(1000);
}
Result
Delete the specified ID face or all faces, and print the corresponding data on the serial port.
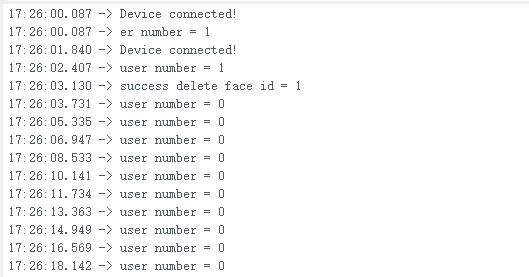
FAQ
For any questions, advice or cool ideas to share, please visit the DFRobot Forum.