Introduction
The SHT4X is the 4th generation digital temperature and humidity sensor from Sensirion. In line with Sensirion’s industry-proven humidity and temperature sensors, the SHT40 offers consistent high accuracy within measuring range.
The SHT40 sensor covers a humidity measurement range of 0 to 100%RH and a temperature detection range of -40°C to 125°C with a typical accuracy of ±1.8%RH and ±0.2℃.
The internal variable power heater enables the device to work properly under extreme operating conditions like condensing environment.
The board supply voltage of 3.3V to 5V and an current consumption below 0.15mA in low power mode make the SHT40 perfectly suitable for mobile or wireless battery-driven applications. It is suitable for urban environment monitoring, intelligent buildings, industrial automation, smart home and other Internet of Things applications.
Features
- High Accuracy
- Low Power Comsumption
- Small Size
- Fast Response
Applications
- Intelligent Buidings and Furniture
- Weather Station
- Warehouses
- Animals and Plants Culture
- Animal Incubator
- Germinating Box for plant seed
Specification
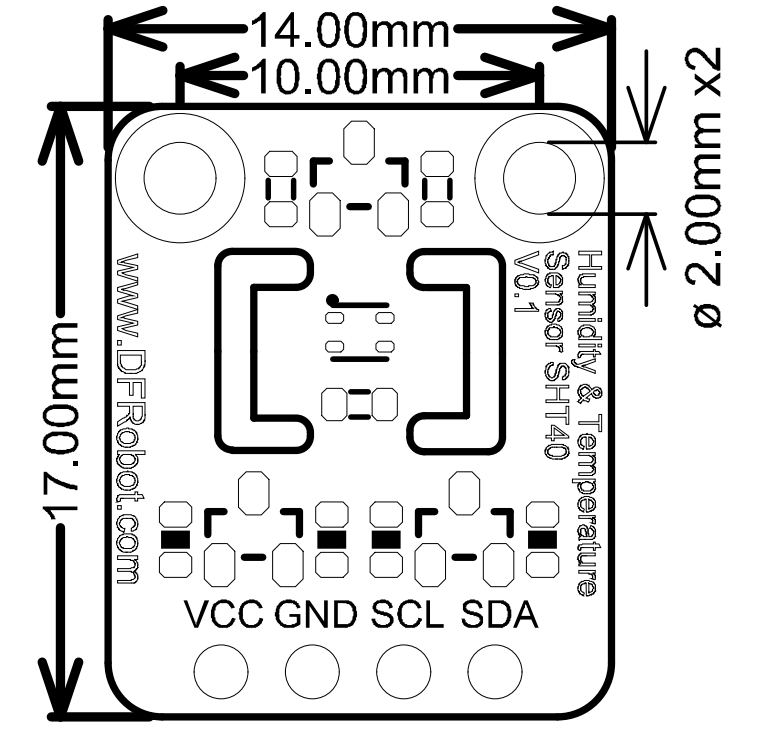
- Operating Voltage: 3.3V-5V
- Operating Current: 0.45mA
- Communication Interface: I2C
- Response Time: 8s (tau63%)
- Humidity Measurement Range: 0~100%RH
- Humidity Measurement Accuracy: ±1.8%
- Temperature Measurement Range: -40~+125℃ (-40 to +275℉)
- Temperature Measurement Accuracy: ±0.2℃
- Dimension: 14×17mm/0.55×0.67”
- Mounting Hole Size: M2 (2mm)
- Mounting Hole Pitch: 10mm
Board Overview
NO. | Silkscreen | Function |
---|---|---|
1 | VCC | Positive Pole |
2 | GND | Negative Pole |
3 | SCL | I2C ClockLine |
4 | SDA | I2C Data Line |
Tutorial
Requirements
- Hardware
- DFRduino UNO R3 (or similar) x 1
- SHTC3 Digital Temperature and Humudity Sensor x 1#
- M-M/F-M/F-F Jumper wires
- Software
- Arduino IDE
- Download and install the SHT Library (About how to install the library?)
- API Functions
/**
* @brief 对主控板的IIC进行了初始化
*/
void begin();
/**
* @brief 获取温度数据
* @return 温度值,单位:摄氏度
*/
float getTemperature();
/**
* @brief 获取湿度数据
* @return 湿度值,单位:%RH
*/
float getHumidity();
/**
* @brief 获取温湿度数据
* @param tem 存放温度数据的引用
* @param hum 存放湿度数据的引用
*/
void getTemHum(float &tem, float &hum);
/**
* @brief 设置传感器工作模式
* @param mode 传感器的工作模式
* @n SHTC3:
* @n PRECISION_HIGH_CLKSTRETCH_ON Clock Stretching Enabled
* @n PRECISION_HIGH_CLKSTRETCH_OFF Clock Stretching Disabled
* @n PRECISION_LOW_CLKSTRETCH_ON Clock Stretching Enabled & Low Power
* @n PRECISION_LOW_CLKSTRETCH_OFF Clock Stretching Disabled & Low Power
* @n SHT40:
* @n PRECISION_HIGH_HEATER_OFF measure T & RH with high precision (high repeatability)
* @n PRECISION_MID_HEATER_OFF measure T & RH with medium precision (medium repeatability)
* @n PRECISION_LOW_HEATER_OFF measure T & RH with lowest precision (low repeatability)
* @n PRECISION_HIGH_HEATER_1S activate highest heater power & high precis. meas. (typ. 200mW @ 3.3V) for 1s
* @n PRECISION_HIGH_HEATER_100MS activate highest heater power & high precis. meas. (typ. 200mW @ 3.3V) for 0.1s
* @n PRECISION_MID_HEATER_1S activate medium heater power & high precis. meas. (typ. 110mW @ 3.3V) for 1s
* @n PRECISION_MID_HEATER_100MS activate medium heater power & high precis. meas. (typ. 110mW @ 3.3V) for 0.1s
* @n PRECISION_LOW_HEATER_1S activate lowest heater power & high precis. meas. (typ. 20mW @ 3.3V) for 1s
* @n PRECISION_LOW_HEATER_100MS activate lowest heater power & high precis. meas. (typ. 20mW @ 3.3V) for 0.1s
*/
void setMode(uint16_t mode) ;
/**
* @brief 获取传感器的唯一标识符
* @return 获取成功返回传感器的唯一标识符,失败返回0
*/
uint32_t getDeviceID();
/**
* @brief software reset
*/
void softwareReset() ;
/**
* @brief Obtain raw data of temperature and humidity
* @param temp Pointer to the address of the original value of the temperature
* @param hun Pointer to the address of the original value of the humidity
* @return Is the data obtained correct? return true The data is correct ; return false The data is incorrect
*/
bool getTandRHRawData(uint16_t *temp, uint16_t *hum);
Connection Diagram
Sample Code - Read Data
Record program and read the current temperature and humidity data.
/*!
* @file temperatureAndHumidity.ino
* @brief Measurement of temperature and humidity
* @n 本传感器可测量温湿度数据,温度的测量范围在-40~125 ℃ ,湿度的测量范围在 0~100 %RH。
* @n 本传感器提供高、中、低,三种测量精度,还提供了高、中、低,三种加热功率对片上加热器进行加热。
* @n 使用加热器的主要情景:
* @n 1、Removal of condensed / spray water on the sensor surface. Although condensed water is not a reliability / quality problem to the sensor, it will however make the sensor nonresponsive to RH changes in the air as long as there is liquid water on the surface.
* @n 2、Creep-free operation in high humid environments. Periodic heating pulses allow for creepSTXfree high-humidity measurements for extended times.
* @n 实验现象 每次1秒钟测量一次温湿度数据并且通过串口打印,当湿度超过80%RH时,启动一次加热器。
* @copyright Copyright (c) 2010 DFRobot Co.Ltd (http://www.dfrobot.com)
* @licence The MIT License (MIT)
* @author [yangfeng]<feng.yang@dfrobot.com>
* @version V1.0
* @date 2021-03-19
* @get from https://www.dfrobot.com
* @url https://github.com/DFRobot/DFRobot_SHT
*/
#include"DFRobot_SHT40.h"
/**
* 对于SHT40传感器这里支持两种型号,第一种是SHT40_AD1B, 第二种是SHT40_BD1B,这两种型号对应不同的IIC设备地址,这里对它们进行了封装,用户可传入如下两种参数
* 型号为SHT40_AD1B的传感器使用: SHT40_AD1B_IIC_ADDR
* 型号为SHT40_AD1B的传感器使用: SHT40_BD1B_IIC_ADDR
*/
DFRobot_SHT40 SHT40(SHT40_AD1B_IIC_ADDR);
uint32_t id = 0;
float temperature, humidity;
void setup() {
Serial.begin(9600);
SHT40.begin();
while((id = SHT40.getDeviceID()) == 0){
Serial.println("ID retrieval error, please check whether the device is connected correctly!!!");
delay(1000);
}
delay(1000);
Serial.print("id :0x"); Serial.println(id, HEX);
}
void loop() {
/**
* mode 用来配置传感器的工作模式
* PRECISION_HIGH measure T & RH with high precision (high repeatability)
* PRECISION_MID measure T & RH with medium precision (medium repeatability)
* PRECISION_LOW measure T & RH with lowest precision (low repeatability)
*/
temperature = SHT40.getTemperature(/*mode = */PRECISION_HIGH);
/**
* mode 用来配置传感器的工作模式
* PRECISION_HIGH measure T & RH with high precision (high repeatability)
* PRECISION_MID measure T & RH with medium precision (medium repeatability)
* PRECISION_LOW measure T & RH with lowest precision (low repeatability)
*/
humidity = SHT40.getHumidity(/*mode = */PRECISION_HIGH);
if(temperature == MODE_ERR){
Serial.println("Incorrect mode configuration to get temperature");
} else{
Serial.print("Temperature :"); Serial.print(temperature); Serial.println(" C");
}
if(humidity == MODE_ERR){
Serial.println("The mode for getting humidity was misconfigured");
} else{
Serial.print("Humidity :"); Serial.print(humidity); Serial.println(" %RH");
}
/**
* mode 用来配置传感器的工作模式
* PRECISION_HIGH measure T & RH with high precision (high repeatability)
* PRECISION_MID measure T & RH with medium precision (medium repeatability)
* PRECISION_LOW measure T & RH with lowest precision (low repeatability)
*/
//if(SHT40.getTemHum(temperature,humidity,PRECISION_HIGH)){
// Serial.print("Temperature :"); Serial.print(temperature); Serial.println(" C");
// Serial.print("Humidity :"); Serial.print(humidity); Serial.println(" %RH");
//} else{
// Serial.println("Pattern configuration error");
//}
if(humidity > 80){
/**
* mode 用来配置传感器的工作模式
* POWER_CONSUMPTION_H_HEATER_1S activate highest heater power & high precis. meas. for 1s
* POWER_CONSUMPTION_H_HEATER_100MS activate highest heater power & high precis. meas. for 0.1s
* POWER_CONSUMPTION_M_HEATER_1S activate medium heater power & high precis. meas. for 1s
* POWER_CONSUMPTION_M_HEATER_100MS activate medium heater power & high precis. meas. for 0.1s
* POWER_CONSUMPTION_L_HEATER_1S activate lowest heater power & high precis. meas. for 1s
* POWER_CONSUMPTION_L_HEATER_100MS activate lowest heater power & high precis. meas. for 0.1s
*/
SHT40.enHeater(/*mode = */POWER_CONSUMPTION_H_HEATER_1S);
}
delay(1000);
Serial.println("----------------------------------------");
}
Expected Results
Open the serial port to display the current temperature and humidity data.
FAQ
For any questions, advice or cool ideas to share, please visit the DFRobot Forum.