Introduction
This is a 1.51”SSD1309 transparent monochrome OLED display(light blue) with a converter,full-view and blue display. For convenience, the small converter comes with two available connections: GDI and SPI. And there are all kinds of display code samples for meeting the basic requirements of most users.
Features
- Transparent OLED display
- Full-view display
- High resolution
- Blue display
Application
- Smart home transformation
- DIY Desktop display
- Interactive display for booth
- 3D Display
Specification
- Screen Interface: 8-bit 68xx/80xx Parallel, 3-/4-wire SPI, 12C
- Adapter Interface: SPI, GDI
- Adapter Size: 18 x 28mm/0.71 x 1.10inch
- Working Voltage: 3.3V
- View Angle: full view
- Driver Chip: SSD1309
- Display Color: blue
- Display Area: 35.05 x 15.32 (mm)/1.40 x 0.60inch
- Pixel Pitch: 0.274 x 0.274mm
- Pixel Size: 0.254 x 0.254mm
- Screen Size: 41.92x27.08mm/1.65 x 1.07inch
- The fully transparent region resolution :128*56
- Working Temperature: -40~70 ℃
Board Overview
Description of pin function
Pin Name | Funtions | |
---|---|---|
1 | VCC | Power(3.3V-5V) |
2 | GND | Negative pole |
3 | SCLK | Clock |
4 | MOSI | Data (sent by host and received by slave) |
5 | CS | Screen piece selected |
6 | RES | Reset |
7 | DC | Data/Command |
Tutorial
The product adopts SPI communication with onboard GDI interface, which greatly reduces the wiring complexity.
Requirements
- Hardware
- ESP32-E (or similar) x 1
- 1.51" Transparent OLED Display Module x1
- Dupont Wires
- Software
- Arduino IDE
- Download and install the U8g2 Arduino Library (About how to install the library?)
Connection Diagram
- Sample Code:
Note:
1.The demos of this product are all stored in the file U8g2_Arduino-master> DFRobot_Demo> 1.51 inch OLED12864-SSD1309.
2.Before burning the Demo, please open the corresponding materialization function (U8G2_SSD1309_128X64_NONAME2_1_4W_HW_SPI u8g2).
Sample Code 1-CommonIcon.ino
/*!
* @file CommonIcon.ino
* @brief Display of several common icons supported in U8G2
* @n U8G2 supports multiple sizes of icons, this demo selects several for display
* @n U8G2 GitHub Link:https://github.com/olikraus/u8g2/wiki/fntlistall
*
* @copyright Copyright (c) 2010 DFRobot Co.Ltd (http://www.dfrobot.com)
* @licence The MIT License (MIT)
* @author [Ivey](Ivey.lu@dfrobot.com)
* @maintainer [Fary](feng.yang@dfrobot.com)
* @version V1.0
* @date 2019-10-15
* @url https://github.com/DFRobot/U8g2_Arduino
*/
#include <Arduino.h>
#include <U8g2lib.h>
#include <SPI.h>
/*
* Display hardware IIC interface constructor
*@param rotation:U8G2_R0 Not rotate, horizontally, draw direction from left to right
U8G2_R1 Rotate clockwise 90 degrees, drawing direction from top to bottom
U8G2_R2 Rotate 180 degrees clockwise, drawing in right-to-left directions
U8G2_R3 Rotate clockwise 270 degrees, drawing direction from bottom to top
U8G2_MIRROR Normal display of mirror content (v2.6.x version used above)
Note: U8G2_MIRROR need to be used with setFlipMode().
*@param reset:U8x8_PIN_NONE Indicates that the pin is empty and no reset pin is used
* Display hardware SPI interface constructor
*@param Just connect the CS pin (pins are optional)
*@param Just connect the DC pin (pins are optional)
*
*/
//U8G2_SSD1306_128X64_NONAME_F_HW_I2C u8g2(/* rotation=*/U8G2_R0, /* reset=*/ U8X8_PIN_NONE); // M0/ESP32/ESP8266/mega2560/Uno/Leonardo
#if defined ARDUINO_SAM_ZERO
#define OLED_DC 7
#define OLED_CS 5
#define OLED_RST 6
/*ESP32 */
#elif defined(ESP32)
#define OLED_DC D2
#define OLED_CS D6
#define OLED_RST D3
/*ESP8266*/
#elif defined(ESP8266)
#define OLED_DC D4
#define OLED_CS D6
#define OLED_RST D5
/*AVR series board*/
#else
#define OLED_DC 2
#define OLED_CS 3
#define OLED_RST 4
#endif
U8G2_SSD1309_128X64_NONAME2_1_4W_HW_SPI u8g2(/* rotation=*/U8G2_R0, /* cs=*/ OLED_CS, /* dc=*/ OLED_DC,/* reset=*/OLED_RST);
void setup(void)
{
u8g2.begin(); //init
u8g2.setFontPosTop(); /**When you use drawStr to display strings, the default criteria is to display the lower-left
* coordinates of the characters.The function can be understood as changing the coordinate position to the upper left
* corner of the display string as the coordinate standard.*/
}
void loop(void)
{
/*
* firstPage will change the current page number position to 0
* When modifications are between firstpage and nextPage, they will be re-rendered at each time.
* This method consumes less ram space than sendBuffer
*/
u8g2.firstPage();
for(int i = 64 ; i <287; i++){
u8g2.clear();
do
{
u8g2.setFont(u8g2_font_open_iconic_all_4x_t ); //Set the font to "u8g2_font_open_iconic_all_4x_t"
/*
* Draw a single character. The character is placed at the specified pixel posion x and y.
* U8g2 supports the lower 16 bit of the unicode character range (plane 0/Basic Multilingual Plane):
* The encoding can be any value from 0 to 65535. The glyph can be drawn only, if the encoding exists in the active font.
*/
u8g2.drawGlyph(/* x=*/4, /* y=*/16, /* encoding=*/i);
u8g2.drawGlyph(/* x=*/44, /* y=*/16, /* encoding=*/i+1);
u8g2.drawGlyph(/* x=*/84, /* y=*/16, /* encoding=*/i+2);
} while ( u8g2.nextPage() );
i = i+3;
delay(2000);
}
}
Result(There are many demo screens in this example. The following are some pictures in the demo screen ):
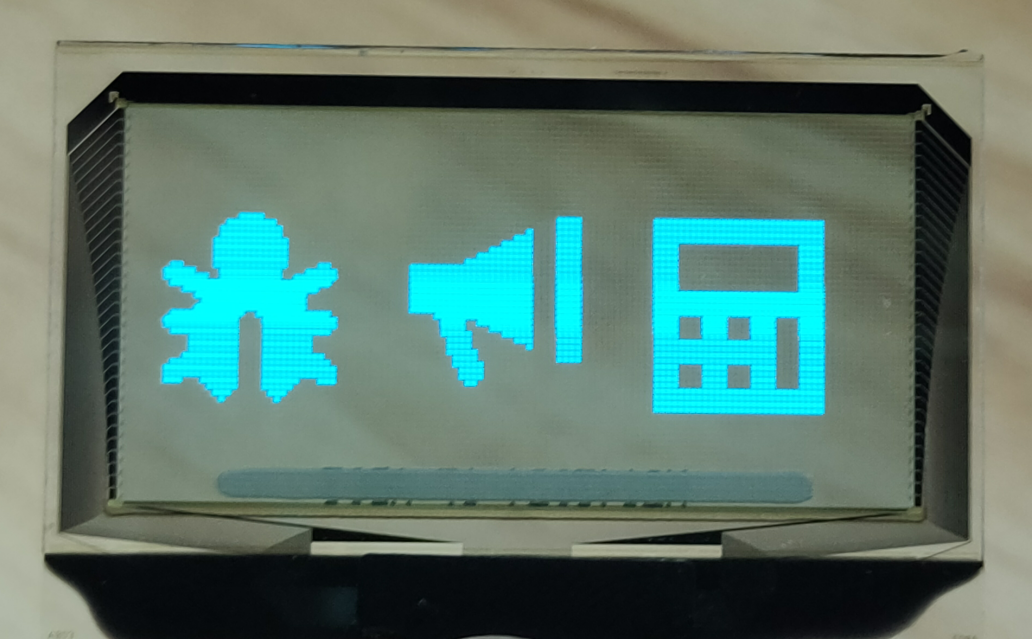
Sample Code 2-Cube.ino
/*!
* @file Cube.ino
* @brief Rotating 3D stereoscopic graphics
* @n This is a simple rotating tetrahexon
*
* @copyright Copyright (c) 2010 DFRobot Co.Ltd (http://www.dfrobot.com)
* @licence The MIT License (MIT)
* @author [Ivey](Ivey.lu@dfrobot.com)
* @maintainer [Fary](feng.yang@dfrobot.com)
* @version V1.0
* @maintainer [Fary](feng.yang@dfrobot.com)
* @version V1.0
* @date 2019-10-15
* @url https://github.com/DFRobot/U8g2_Arduino
*/
#include <Arduino.h>
#include <U8g2lib.h>
#include <SPI.h>
/*
* Display hardware IIC interface constructor
*@param rotation:U8G2_R0 Not rotate, horizontally, draw direction from left to right
U8G2_R1 Rotate clockwise 90 degrees, drawing direction from top to bottom
U8G2_R2 Rotate 180 degrees clockwise, drawing in right-to-left directions
U8G2_R3 Rotate clockwise 270 degrees, drawing direction from bottom to top
U8G2_MIRROR Normal display of mirror content (v2.6.x version used above)
Note: U8G2_MIRROR need to be used with setFlipMode().
*@param reset:U8x8_PIN_NONE Indicates that the pin is empty and no reset pin is used
* Display hardware SPI interface constructor
*@param Just connect the CS pin (pins are optional)
*@param Just connect the DC pin (pins are optional)
*
*/
#if defined ARDUINO_SAM_ZERO
#define OLED_DC 7
#define OLED_CS 5
#define OLED_RST 6
/*ESP32 */
#elif defined(ESP32)
#define OLED_DC D2
#define OLED_CS D6
#define OLED_RST D3
/*ESP8266*/
#elif defined(ESP8266)
#define OLED_DC D4
#define OLED_CS D6
#define OLED_RST D5
/*AVR series board*/
#else
#define OLED_DC 2
#define OLED_CS 3
#define OLED_RST 4
#endif
U8G2_SSD1309_128X64_NONAME2_1_4W_HW_SPI u8g2(/* rotation=*/U8G2_R0, /* cs=*/ OLED_CS, /* dc=*/ OLED_DC,/* reset=*/OLED_RST);
//2D array: The coordinates of all vertices of the tetrahesome are stored
double tetrahedron[4][3] = {{0,20,-20},{-20,-20,-20},{20,-20,-20},{0,0,20}};
void setup(void) {
u8g2.begin();
}
void loop(void) {
/*
* firstPage will change the current page number position to 0
* When modifications are between firstpage and nextPage, they will be re-rendered at each time.
* This method consumes less ram space than sendBuffer
*/
u8g2.firstPage();
do {
//Connect the corresponding points inside the tetrahethal together
u8g2.drawLine(OxyzToOu(tetrahedron[0][0], tetrahedron[0][2]), OxyzToOv(tetrahedron[0][1], tetrahedron[0][2]), OxyzToOu(tetrahedron[1][0], tetrahedron[1][2]), OxyzToOv(tetrahedron[1][1], tetrahedron[1][2]));
u8g2.drawLine(OxyzToOu(tetrahedron[1][0], tetrahedron[1][2]), OxyzToOv(tetrahedron[1][1], tetrahedron[1][2]), OxyzToOu(tetrahedron[2][0], tetrahedron[2][2]), OxyzToOv(tetrahedron[2][1], tetrahedron[2][2]));
u8g2.drawLine(OxyzToOu(tetrahedron[0][0], tetrahedron[0][2]), OxyzToOv(tetrahedron[0][1], tetrahedron[0][2]), OxyzToOu(tetrahedron[2][0], tetrahedron[2][2]), OxyzToOv(tetrahedron[2][1], tetrahedron[2][2]));
u8g2.drawLine(OxyzToOu(tetrahedron[0][0], tetrahedron[0][2]), OxyzToOv(tetrahedron[0][1], tetrahedron[0][2]), OxyzToOu(tetrahedron[3][0], tetrahedron[3][2]), OxyzToOv(tetrahedron[3][1], tetrahedron[3][2]));
u8g2.drawLine(OxyzToOu(tetrahedron[1][0], tetrahedron[1][2]), OxyzToOv(tetrahedron[1][1], tetrahedron[1][2]), OxyzToOu(tetrahedron[3][0], tetrahedron[3][2]), OxyzToOv(tetrahedron[3][1], tetrahedron[3][2]));
u8g2.drawLine(OxyzToOu(tetrahedron[2][0], tetrahedron[2][2]), OxyzToOv(tetrahedron[2][1], tetrahedron[2][2]), OxyzToOu(tetrahedron[3][0], tetrahedron[3][2]), OxyzToOv(tetrahedron[3][1], tetrahedron[3][2]));
// Rotate 0.1°
rotate(0.1);
} while ( u8g2.nextPage() );
//delay(50);
}
/*!
* @brief Convert xz in the three-dimensional coordinate system Oxyz
* into the u coordinate inside the two-dimensional coordinate system Ouv
* @param x in Oxyz
* @param z in Oxyz
* @return u in Ouv
*/
int OxyzToOu(double x,double z){
return (int)((x + 64) - z*0.35);
}
/*!
* @brief Convert the yz in the three-dimensional coordinate system Oxyz into the v coordinate inside
* the two-dimensional coordinate system Ouv
* @param y in Oxyz
* @param z in Oxyz
* @return v in Ouv
*/
int OxyzToOv(double y,double z){
return (int)((y + 26) - z*0.35);
}
/*!
* @brief Rotate the coordinates of all points of the entire 3D graphic around the Z axis
* @param angle represents the angle to rotate
*
* z rotation (z unchanged)
x3 = x2 * cosb - y1 * sinb
y3 = y1 * cosb + x2 * sinb
z3 = z2
*/
void rotate(double angle)
{
double rad, cosa, sina, Xn, Yn;
rad = angle * PI / 180;
cosa = cos(rad);
sina = sin(rad);
for (int i = 0; i < 4; i++)
{
Xn = (tetrahedron[i][0] * cosa) - (tetrahedron[i][1] * sina);
Yn = (tetrahedron[i][0] * sina) + (tetrahedron[i][1] * cosa);
//Store converted coordinates into an array of coordinates
//Because it rotates around the Z-axis, the coordinates of the point z-axis remain unchanged
tetrahedron[i][0] = Xn;
tetrahedron[i][1] = Yn;
}
}
Result:

Sample Code 3-Font.ino
/*!
* @file Font.ino
* @brief Show several U8G2-supported fonts
* @n U8G2 supports multiple fonts, and this demo is just a few fonts to display
* @n U8G2 Font GitHub Link:https://github.com/olikraus/u8g2/wiki/fntlistall
*
* @copyright Copyright (c) 2010 DFRobot Co.Ltd (http://www.dfrobot.com)
* @licence The MIT License (MIT)
* @author [Ivey](Ivey.lu@dfrobot.com)
* @maintainer [Fary](feng.yang@dfrobot.com)
* @version V1.0
* @date 2019-10-15
* @url https://github.com/DFRobot/U8g2_Arduino
*/
#include <Arduino.h>
#include <U8g2lib.h>
#include <SPI.h>
/*
* Display hardware IIC interface constructor
*@param rotation:U8G2_R0 Not rotate, horizontally, draw direction from left to right
U8G2_R1 Rotate clockwise 90 degrees, drawing direction from top to bottom
U8G2_R2 Rotate 180 degrees clockwise, drawing in right-to-left directions
U8G2_R3 Rotate clockwise 270 degrees, drawing direction from bottom to top
U8G2_MIRROR Normal display of mirror content (v2.6.x version used above)
Note: U8G2_MIRROR need to be used with setFlipMode().
*@param reset:U8x8_PIN_NONE Indicates that the pin is empty and no reset pin is used
* Display hardware SPI interface constructor
*@param Just connect the CS pin (pins are optional)
*@param Just connect the DC pin (pins are optional)
*
*/
#if defined ARDUINO_SAM_ZERO
#define OLED_DC 7
#define OLED_CS 5
#define OLED_RST 6
/*ESP32 */
#elif defined(ESP32)
#define OLED_DC D2
#define OLED_CS D6
#define OLED_RST D3
/*ESP8266*/
#elif defined(ESP8266)
#define OLED_DC D4
#define OLED_CS D6
#define OLED_RST D5
/*AVR series board*/
#else
#define OLED_DC 2
#define OLED_CS 3
#define OLED_RST 4
#endif
U8G2_SSD1309_128X64_NONAME2_1_4W_HW_SPI u8g2(/* rotation=*/U8G2_R0, /* cs=*/ OLED_CS, /* dc=*/ OLED_DC,/* reset=*/OLED_RST);
void setup(void)
{
u8g2.begin(); //Initialize the function
u8g2.setFontPosTop(); /**When you use drawStr to display strings, the default criteria is to display the lower-left
* coordinates of the characters.The function can be understood as changing the coordinate position to the upper left
* corner of the display string as the coordinate standard.*/
}
void draw(int a )
{
switch(a)
{
case 0:
u8g2.setFont(u8g2_font_bubble_tr ); //Set the font set, which is“u8g2_font_bubble_tr“
u8g2.drawStr(/* x=*/0,/* y=*/0, "DFR123"); //Start drawing strings at the coordinates of x-0, y-0 “DFR123”
u8g2.setFont(u8g2_font_lucasarts_scumm_subtitle_o_tf );
u8g2.drawStr(0, 25, "DFR123");
u8g2.setFont(u8g2_font_HelvetiPixelOutline_tr );
u8g2.drawStr(0, 45, "DFR123");
break;
case 1:
u8g2.setFont(u8g2_font_tenstamps_mr );
u8g2.drawStr(0,0, "DFR123");
u8g2.setFont(u8g2_font_jinxedwizards_tr );
u8g2.drawStr(56, 16, "DFR123");
u8g2.setFont(u8g2_font_secretaryhand_tr );
u8g2.drawStr(0, 32, "DFR123");
u8g2.setFont(u8g2_font_freedoomr10_mu );
u8g2.drawStr(56, 48, "DFR123");
break;
}
}
void loop()
{
for( int i = 0; i <2 ; i++)
{
/*
* firstPage will change the current page number position to 0
* When modifications are between firstpage and nextPage, they will be re-rendered at each time.
* This method consumes less ram space than sendBuffer
*/
u8g2.firstPage();
do
{
draw(i);
} while( u8g2.nextPage() );
delay(2000);
}
}
Result:
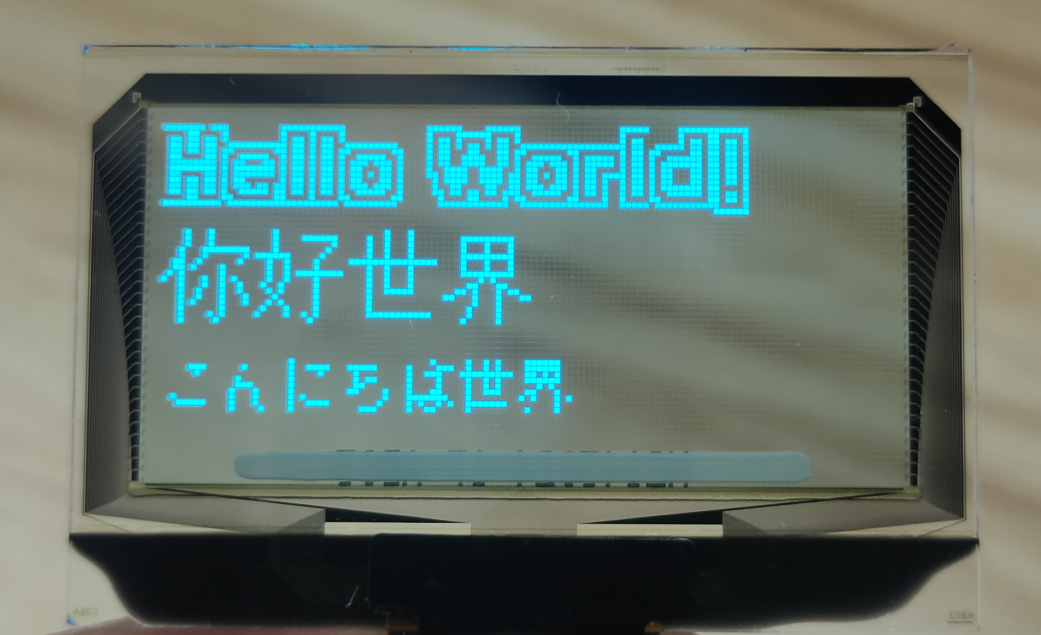
Sample Code 4-FontDirection.ino
/*!
* @file FontDirection.ino
* @brief Display of several font directions supported in U8G2
* @n U8G2 supports multiple fonts, and this demo shows only four directions (no mirrors are shown).
* @n U8G2 Font GitHub Link:https://github.com/olikraus/u8g2/wiki/fntlistall
*
* @copyright Copyright (c) 2010 DFRobot Co.Ltd (http://www.dfrobot.com)
* @licence The MIT License (MIT)
* @author [Ivey](Ivey.lu@dfrobot.com)
* @maintainer [Fary](feng.yang@dfrobot.com)
* @version V1.0
* @date 2019-10-15
* @url https://github.com/DFRobot/U8g2_Arduino
*/
#include <Arduino.h>
#include <U8g2lib.h>
#include <SPI.h>
/*
* Display hardware IIC interface constructor
*@param rotation:U8G2_R0 Not rotate, horizontally, draw direction from left to right
U8G2_R1 Rotate clockwise 90 degrees, drawing direction from top to bottom
U8G2_R2 Rotate 180 degrees clockwise, drawing in right-to-left directions
U8G2_R3 Rotate clockwise 270 degrees, drawing direction from bottom to top
U8G2_MIRROR Normal display of mirror content (v2.6.x version used above)
Note: U8G2_MIRROR need to be used with setFlipMode().
*@param reset:U8x8_PIN_NONE Indicates that the pin is empty and no reset pin is used
* Display hardware SPI interface constructor
*@param Just connect the CS pin (pins are optional)
*@param Just connect the DC pin (pins are optional)
*
*/
#if defined ARDUINO_SAM_ZERO
#define OLED_DC 7
#define OLED_CS 5
#define OLED_RST 6
/*ESP32 */
#elif defined(ESP32)
#define OLED_DC D2
#define OLED_CS D6
#define OLED_RST D3
/*ESP8266*/
#elif defined(ESP8266)
#define OLED_DC D4
#define OLED_CS D6
#define OLED_RST D5
/*AVR series board*/
#else
#define OLED_DC 2
#define OLED_CS 3
#define OLED_RST 4
#endif
U8G2_SSD1309_128X64_NONAME2_1_4W_HW_SPI u8g2(/* rotation=*/U8G2_R0, /* cs=*/ OLED_CS, /* dc=*/ OLED_DC,/* reset=*/OLED_RST);
//width:30,height:30
const uint8_t col[] U8X8_PROGMEM= {0x00,0xc0,0x00,0x00,0x00,0xe0,0x01,0x00,0x00,0xe0,0x01,0x00,0x00,
0xc0,0x00,0x00,0x00,0xc0,0x00,0x00,0x00,0xc0,0x00,0x00,0x00,0xe0,
0x01,0x00,0x00,0xf8,0x07,0x00,0x06,0xfe,0x1f,0x18,0x07,0xff,0x3f,
0x38,0xdf,0xff,0xff,0x3e,0xfa,0xff,0xff,0x17,0xf0,0xff,0xff,0x03,
0xe0,0xff,0xff,0x01,0xe0,0xff,0xff,0x01,0xe0,0xff,0xff,0x01,0xe0,
0xff,0xff,0x01,0x20,0x00,0x00,0x01,0xa0,0xff,0x7f,0x01,0xa0,0x01,
0x60,0x01,0x20,0x07,0x38,0x01,0xe0,0x0c,0xcc,0x01,0xe0,0x39,0xe7,
0x01,0xe0,0xe7,0xf9,0x01,0xc0,0x1f,0xfe,0x00,0x80,0xff,0x7f,0x00,
0x00,0xfe,0x1f,0x00,0x00,0xf8,0x07,0x00,0x00,0xe0,0x01,0x00,0x00,
0xc0,0x00,0x00};
void setup() {
u8g2.begin();
u8g2.setFontPosTop();/**When you use drawStr to display strings, the default criteria is to display the lower-left
* coordinates of the characters.The function can be understood as changing the coordinate position to the upper left
* corner of the display string as the coordinate standard.*/
}
void Rotation() {
u8g2.setFont(u8g2_font_bracketedbabies_tr);
u8g2.firstPage();
do {
u8g2.drawXBMP( /* x=*/0 , /* y=*/0 , /* width=*/30 , /* height=*/30 , col );//Draw a XBM Bitmap. Position (x,y) is the upper left corner of the bitmap. XBM contains monochrome, 1-bit bitmaps.
/*@brief Set the drawing direction of all strings or glyphs setFontDirection(uint8_t dir)
*@param dir=0
dir=1, rotate 0°
dir=2, rotate 180°
dir=3, rotate 270°
*/
u8g2.setFontDirection(0);
u8g2.drawStr( /* x=*/64,/* y=*/32, " DFR"); //Start drawing strings at the coordinates of x-64, y-32 “DFR”
u8g2.setFontDirection(1);
u8g2.drawStr(64,32, " DFR");
u8g2.setFontDirection(2);
u8g2.drawStr(64,32, " DFR");
u8g2.setFontDirection(3);
u8g2.drawStr(64,32, " DFR");
}while(u8g2.nextPage());
delay(1500);
}
void loop(void)
{
u8g2.setFont( u8g2_font_sticker_mel_tr);
for(int i = 0 ; i < 4 ; i++ )
{
switch(i)
{
case 0:
u8g2.setFontDirection(0);
break;
case 1:
u8g2.setFontDirection(1);
break;
case 2:
u8g2.setFontDirection(2);
break;
case 3:
u8g2.setFontDirection(3);
break;
}
/*
* firstPage will change the current page number position to 0
* When modifications are between firstpage and nextPage, they will be re-rendered at each time.
* This method consumes less ram space than sendBuffer
*/
u8g2.firstPage();
do
{
u8g2.drawStr(64, 32, "DFR");
u8g2.drawXBMP( 0 , 0 , 30 , 30 , col );
}while(u8g2.nextPage());
delay(500);
}
Rotation();
}
Result:
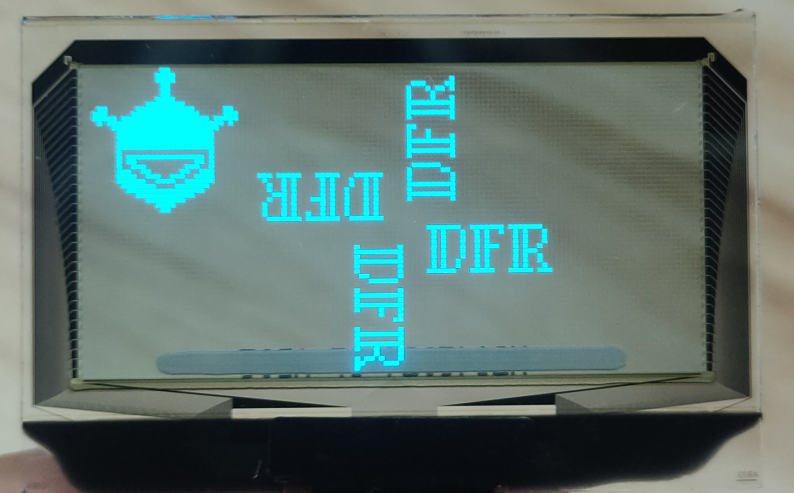
Sample Code 5-GraphicsTest.ino
/*
GraphicsTest.ino
Universal 8bit Graphics Library (https://github.com/olikraus/u8g2/)
Copyright (c) 2016, olikraus@gmail.com
All rights reserved.
Redistribution and use in source and binary forms, with or without modification,
are permitted provided that the following conditions are met:
* Redistributions of source code must retain the above copyright notice, this list
of conditions and the following disclaimer.
* Redistributions in binary form must reproduce the above copyright notice, this
list of conditions and the following disclaimer in the documentation and/or other
materials provided with the distribution.
THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND
CONTRIBUTORS "AS IS" AND ANY EXPRESS OR IMPLIED WARRANTIES,
INCLUDING, BUT NOT LIMITED TO, THE IMPLIED WARRANTIES OF
MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE
DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR
CONTRIBUTORS BE LIABLE FOR ANY DIRECT, INDIRECT, INCIDENTAL,
SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT
NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES;
LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER
CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT,
STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE)
ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF
ADVISED OF THE POSSIBILITY OF SUCH DAMAGE.
*/
#include <Arduino.h>
#include <U8g2lib.h>
#include <SPI.h>
/*
U8g2lib Example Overview:
Frame Buffer Examples: clearBuffer/sendBuffer. Fast, but may not work with all Arduino boards because of RAM consumption
Page Buffer Examples: firstPage/nextPage. Less RAM usage, should work with all Arduino boards.
U8x8 Text Only Example: No RAM usage, direct communication with display controller. No graphics, 8x8 Text only.
This is a page buffer example.
*/
// Please UNCOMMENT one of the contructor lines below
// U8g2 Contructor List (Picture Loop Page Buffer)
// The complete list is available here: https://github.com/olikraus/u8g2/wiki/u8g2setupcpp
// Please update the pin numbers according to your setup. Use U8X8_PIN_NONE if the reset pin is not connected
#if defined ARDUINO_SAM_ZERO
#define OLED_DC 7
#define OLED_CS 5
#define OLED_RST 6
/*ESP32 */
#elif defined(ESP32)
#define OLED_DC D2
#define OLED_CS D6
#define OLED_RST D3
/*ESP8266*/
#elif defined(ESP8266)
#define OLED_DC D4
#define OLED_CS D6
#define OLED_RST D5
/*AVR series board*/
#else
#define OLED_DC 2
#define OLED_CS 3
#define OLED_RST 4
#endif
U8G2_SSD1309_128X64_NONAME2_1_4W_HW_SPI u8g2(/* rotation=*/U8G2_R0, /* cs=*/ OLED_CS, /* dc=*/ OLED_DC,/* reset=*/OLED_RST);
// End of constructor list
void u8g2_prepare(void) {
u8g2.setFont(u8g2_font_6x10_tf); //Set the font to "u8g2_font_6x10_tf"
u8g2.setFontRefHeightExtendedText(); //Ascent will be the largest ascent of "A", "1" or "(" of the current font. Descent will be the descent of "g" or "(" of the current font.
u8g2.setDrawColor(1); //Ascent will be the largest ascent of "A", "1" or "(" of the current font. Descent will be the descent of "g" or "(" of the current font.
u8g2.setFontPosTop(); /*When you use drawStr to display strings, the default criteria is to display the lower-left coordinates of the characters.
XXXX */
u8g2.setFontDirection(0); //Set the screen orientation: 0 -- for normal display
}
void u8g2_box_title(uint8_t a) {
u8g2.drawStr( 10+a*2, 5, "U8g2"); //Draw string "U8g2"
u8g2.drawStr( 10, 20, "GraphicsTest");
u8g2.drawFrame(0,0,u8g2.getDisplayWidth(),u8g2.getDisplayHeight() ); //Start drawing an empty box of width w and height h at a coordinate of (0,0)
}
/*
* Draw solid squares and hollow squares
*/
void u8g2_box_frame(uint8_t a) {
u8g2.drawStr( 0, 0, "drawBox");
u8g2.drawBox(5,10,20,10); //Start drawing a solid square with a width w and a height h at a position with coordinates of (5, 10)
u8g2.drawBox(10+a,15,30,7);
u8g2.drawStr( 0, 30, "drawFrame");
u8g2.drawFrame(5,10+30,20,10);
u8g2.drawFrame(10+a,15+30,30,7);
}
/*
* Draw solid and hollow circles
*/
void u8g2_disc_circle(uint8_t a) {
u8g2.drawStr( 0, 0, "drawDisc");
u8g2.drawDisc(10,18,9); //Draw a solid circle with a radius of 10 at the position (10, 18)
u8g2.drawDisc(24+a,16,7);
u8g2.drawStr( 0, 30, "drawCircle");
u8g2.drawCircle(10,18+30,9); //Draw a hollow circle with a radius of 10 at the position (10, 18+30)
u8g2.drawCircle(24+a,16+30,7);
}
/*
* Draw solid and hollow boxes
*/
void u8g2_r_frame(uint8_t a) {
u8g2.drawStr( 0, 0, "drawRFrame/Box");
u8g2.drawRFrame(5, 10,40,30, a+1); //At the position (5 ,10) start drawing with a width of 40 and a height of 30 a frame with a radius of a+1 circular edge (hollow).
u8g2.drawRBox(50, 10,25,40, a+1); //At the position (50,10) start drawing with a width of 25 and a height of 40 a frame with a circular edge with a radius of a 1 (solid).
}
void u8g2_string(uint8_t a) {
/*@brief Set the drawing direction of all strings or glyphs setFontDirection(uint8_t dir)
*@param dir=0
dir=1, rotate 0°
dir=2, rotate 180°
dir=3, rotate 270°
*/
u8g2.setFontDirection(0);
u8g2.drawStr(30+a,31, " 0");
u8g2.setFontDirection(1);
u8g2.drawStr(30,31+a, " 90");
u8g2.setFontDirection(2);
u8g2.drawStr(30-a,31, " 180");
u8g2.setFontDirection(3);
u8g2.drawStr(30,31-a, " 270");
}
/*
* Draw segments
*/
void u8g2_line(uint8_t a) {
u8g2.drawStr( 0, 0, "drawLine");
u8g2.drawLine(7+a, 10, 40, 55); //Draw a line between two points. (Argument is two-point coordinates)
u8g2.drawLine(7+a*2, 10, 60, 55);
u8g2.drawLine(7+a*3, 10, 80, 55);
u8g2.drawLine(7+a*4, 10, 100, 55);
}
/*
* Draw solid triangles and hollow triangles
*/
void u8g2_triangle(uint8_t a) {
uint16_t offset = a;
u8g2.drawStr( 0, 0, "drawTriangle");
u8g2.drawTriangle(14,7, 45,30, 10,40); //Draw a triangle (solid polygon). (Argument is triangle three vertex coordinates)
u8g2.drawTriangle(14+offset,7-offset, 45+offset,30-offset, 57+offset,10-offset);
u8g2.drawTriangle(57+offset*2,10, 45+offset*2,30, 86+offset*2,53);
u8g2.drawTriangle(10+offset,40+offset, 45+offset,30+offset, 86+offset,53+offset);
}
/*
* Show characters in the ASCII code table
*/
void u8g2_ascii_1() {
char s[2] = " ";
uint8_t x, y;
u8g2.drawStr( 0, 0, "ASCII page 1");
for( y = 0; y < 6; y++ ) {
for( x = 0; x < 16; x++ ) {
s[0] = y*16 + x + 32;
u8g2.drawStr(x*7, y*10+10, s);
}
}
}
void u8g2_ascii_2() {
char s[2] = " ";
uint8_t x, y;
u8g2.drawStr( 0, 0, "ASCII page 2");
for( y = 0; y < 6; y++ ) {
for( x = 0; x < 16; x++ ) {
s[0] = y*16 + x + 160;
u8g2.drawStr(x*7, y*10+10, s);
}
}
}
/*
* Draw a string icon in a UTF-8 encoding
*/
void u8g2_extra_page(uint8_t a)
{
u8g2.drawStr( 0, 0, "Unicode");
u8g2.setFont(u8g2_font_unifont_t_symbols);
u8g2.setFontPosTop();
u8g2.drawUTF8(0, 24, "☀ ☁"); //Start drawing a string icon encoded as UTF-8 at the location (0,24)
switch(a) {
case 0:
case 1:
case 2:
case 3:
u8g2.drawUTF8(a*3, 36, "☂");
break;
case 4:
case 5:
case 6:
case 7:
u8g2.drawUTF8(a*3, 36, "☔");
break;
}
}
/*
* Show the reverse display of font. Which means the font is displayed interchangeable with
* the background color. (For example, it would have been black on white, replaced by white
* on black)
*/
void u8g2_xor(uint8_t a) {
uint8_t i;
u8g2.drawStr( 0, 0, "XOR");
u8g2.setFontMode(1);
u8g2.setDrawColor(2);
for( i = 0; i < 5; i++)
{
u8g2.drawBox(10+i*16, 18 + (i&1)*4, 21,31);
}
u8g2.drawStr( 5+a, 19, "XOR XOR XOR XOR");
u8g2.setDrawColor(0);
u8g2.drawStr( 5+a, 29, "CLR CLR CLR CLR");
u8g2.setDrawColor(1);
u8g2.drawStr( 5+a, 39, "SET SET SET SET");
u8g2.setFontMode(0);
}
/*
*This is the data of converting a graph to bitmap for using the drawXBMP () to display.
*/
#define cross_width 24
#define cross_height 24
static const unsigned char cross_bits[] U8X8_PROGMEM = {
0x00, 0x18, 0x00, 0x00, 0x24, 0x00, 0x00, 0x24, 0x00, 0x00, 0x42, 0x00,
0x00, 0x42, 0x00, 0x00, 0x42, 0x00, 0x00, 0x81, 0x00, 0x00, 0x81, 0x00,
0xC0, 0x00, 0x03, 0x38, 0x3C, 0x1C, 0x06, 0x42, 0x60, 0x01, 0x42, 0x80,
0x01, 0x42, 0x80, 0x06, 0x42, 0x60, 0x38, 0x3C, 0x1C, 0xC0, 0x00, 0x03,
0x00, 0x81, 0x00, 0x00, 0x81, 0x00, 0x00, 0x42, 0x00, 0x00, 0x42, 0x00,
0x00, 0x42, 0x00, 0x00, 0x24, 0x00, 0x00, 0x24, 0x00, 0x00, 0x18, 0x00, };
#define cross_fill_width 24
#define cross_fill_height 24
static const unsigned char cross_fill_bits[] U8X8_PROGMEM = {
0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x18, 0x00, 0x18, 0x64, 0x00, 0x26,
0x84, 0x00, 0x21, 0x08, 0x81, 0x10, 0x08, 0x42, 0x10, 0x10, 0x3C, 0x08,
0x20, 0x00, 0x04, 0x40, 0x00, 0x02, 0x80, 0x00, 0x01, 0x80, 0x18, 0x01,
0x80, 0x18, 0x01, 0x80, 0x00, 0x01, 0x40, 0x00, 0x02, 0x20, 0x00, 0x04,
0x10, 0x3C, 0x08, 0x08, 0x42, 0x10, 0x08, 0x81, 0x10, 0x84, 0x00, 0x21,
0x64, 0x00, 0x26, 0x18, 0x00, 0x18, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, };
#define cross_block_width 14
#define cross_block_height 14
static const unsigned char cross_block_bits[] U8X8_PROGMEM = {
0xFF, 0x3F, 0x01, 0x20, 0x01, 0x20, 0x01, 0x20, 0x01, 0x20, 0x01, 0x20,
0xC1, 0x20, 0xC1, 0x20, 0x01, 0x20, 0x01, 0x20, 0x01, 0x20, 0x01, 0x20,
0x01, 0x20, 0xFF, 0x3F, };
/*
* Draw a bitmap
*/
void u8g2_bitmap_overlay(uint8_t a) {
uint8_t frame_size = 28;
u8g2.drawStr(0, 0, "Bitmap overlay");
u8g2.drawStr(0, frame_size + 12, "Solid / transparent");
u8g2.setBitmapMode(false /* solid */);
u8g2.drawFrame(0, 10, frame_size, frame_size);
u8g2.drawXBMP(2, 12, cross_width, cross_height, cross_bits);
if(a & 4)
u8g2.drawXBMP(7, 17, cross_block_width, cross_block_height, cross_block_bits);
/*
* Set the pattern of the bitmap to define whether the background color is written to the bitmap function
* (Mode0/solid,is_transparent = 0).
* Or not write the background color to the bitmap function. (Mode1/solid,is_transparent = 1).
* The default mode is 0(fixed mode).
*/
u8g2.setBitmapMode(true /* transparent*/);
u8g2.drawFrame(frame_size + 5, 10, frame_size, frame_size);
/*
* The position (x,y) is the upper-left corner of the bitmap. XBM contains a monochrome 1-bit bitmap.
* The current color index is used for drawing (refers to setColorIndex) pixel value1.
*/
u8g2.drawXBMP(frame_size + 7, 12, cross_width, cross_height, cross_bits);
if(a & 4)
u8g2.drawXBMP(frame_size + 12, 17, cross_block_width, cross_block_height, cross_block_bits);
}
void u8g2_bitmap_modes(uint8_t transparent) {
const uint8_t frame_size = 24;
u8g2.drawBox(0, frame_size * 0.5, frame_size * 5, frame_size);
u8g2.drawStr(frame_size * 0.5, 50, "Black");
u8g2.drawStr(frame_size * 2, 50, "White");
u8g2.drawStr(frame_size * 3.5, 50, "XOR");
if(!transparent) {
u8g2.setBitmapMode(false /* solid */);
u8g2.drawStr(0, 0, "Solid bitmap");
} else {
u8g2.setBitmapMode(true /* transparent*/);
u8g2.drawStr(0, 0, "Transparent bitmap");
}
u8g2.setDrawColor(0);// Black
u8g2.drawXBMP(frame_size * 0.5, 24, cross_width, cross_height, cross_bits);//绘制位图
u8g2.setDrawColor(1); // White
u8g2.drawXBMP(frame_size * 2, 24, cross_width, cross_height, cross_bits);
u8g2.setDrawColor(2); // XOR
u8g2.drawXBMP(frame_size * 3.5, 24, cross_width, cross_height, cross_bits);
}
//Define the initial variable for the drawing state
uint8_t draw_state = 0;
/*
* Draw functions: call other functions in an orderly manner.
*/
void draw(void) {
u8g2_prepare();
switch(draw_state >> 3) {
case 0: u8g2_box_title(draw_state&7); break;
case 1: u8g2_box_frame(draw_state&7); break;
case 2: u8g2_disc_circle(draw_state&7); break;
case 3: u8g2_r_frame(draw_state&7); break;
case 4: u8g2_string(draw_state&7); break;
case 5: u8g2_line(draw_state&7); break;
case 6: u8g2_triangle(draw_state&7); break;
case 7: u8g2_ascii_1(); break;
case 8: u8g2_ascii_2(); break;
case 9: u8g2_extra_page(draw_state&7); break;
case 10: u8g2_xor(draw_state&7); break;
case 11: u8g2_bitmap_modes(0); break;
case 12: u8g2_bitmap_modes(1); break;
case 13: u8g2_bitmap_overlay(draw_state&7); break;
}
}
void setup(void) {
u8g2.begin(); //Initialize the function
}
void loop(void) {
// picture loop
u8g2.firstPage();
do {
draw();
} while( u8g2.nextPage() );
// increase the state
draw_state++;
if ( draw_state >= 14*8 )
draw_state = 0;
// delay between each page
delay(150);
}
Result(There are many demo screens in this example. The following are some pictures in the demo screen ):
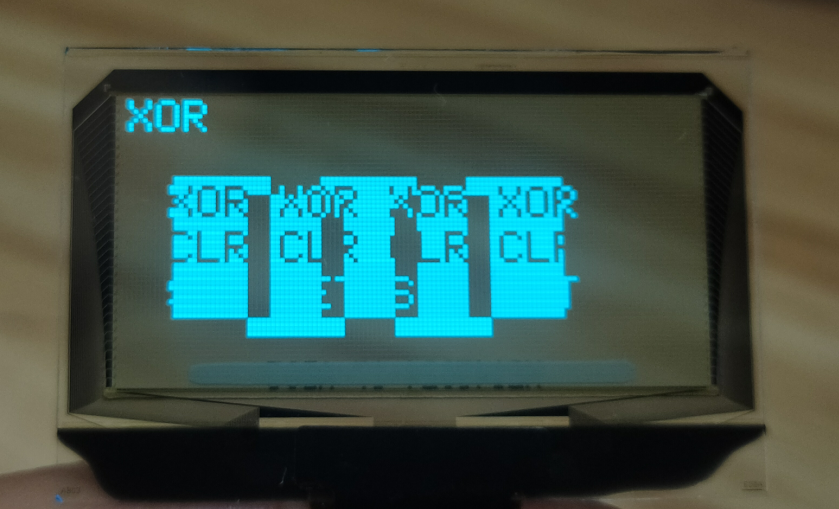
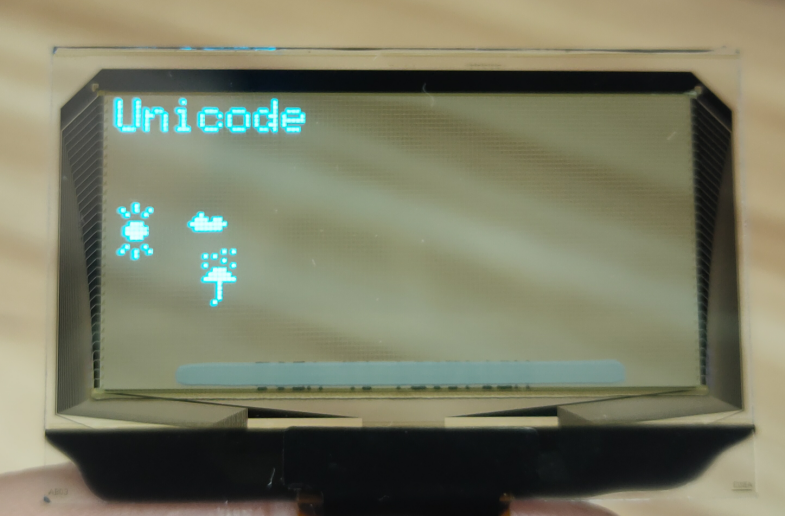
Sample Code 6-HelloWorld.ino
/*!
* @file HelloWorld.ino
* @brief Display Hello World!
* @n U8G2 supports fonts of various sizes. This demo only present one font size to show "Hello World!"
* @n U8G2 Font GitHub link:https://github.com/olikraus/u8g2/wiki/fntlistall
*
* @copyright Copyright (c) 2010 DFRobot Co.Ltd (http://www.dfrobot.com)
* @licence The MIT License (MIT)
* @author [Ivey](Ivey.lu@dfrobot.com)
* @maintainer [Fary](feng.yang@dfrobot.com)
* @version V1.0
* @date 2019-10-15
* @url https://github.com/DFRobot/U8g2_Arduino
*/
#include <Arduino.h>
#include <U8g2lib.h>
#include <SPI.h>
/*
* Display hardware IIC interface constructor
*@param rotation:U8G2_R0 Not rotate, horizontally, draw direction from left to right
U8G2_R1 Rotate clockwise 90 degrees, drawing direction from top to bottom
U8G2_R2 Rotate 180 degrees clockwise, drawing in right-to-left directions
U8G2_R3 Rotate clockwise 270 degrees, drawing direction from bottom to top
U8G2_MIRROR Normal display of mirror content (v2.6.x version used above)
Note: U8G2_MIRROR need to be used with setFlipMode().
*@param reset:U8x8_PIN_NONE Indicates that the pin is empty and no reset pin is used
* Display hardware SPI interface constructor
*@param Just connect the CS pin (pins are optional)
*@param Just connect the DC pin (pins are optional)
*
*/
#if defined ARDUINO_SAM_ZERO
#define OLED_DC 7
#define OLED_CS 5
#define OLED_RST 6
/*ESP32 */
#elif defined(ESP32)
#define OLED_DC D2
#define OLED_CS D6
#define OLED_RST D3
/*ESP8266*/
#elif defined(ESP8266)
#define OLED_DC D4
#define OLED_CS D6
#define OLED_RST D5
/*AVR series board*/
#else
#define OLED_DC 2
#define OLED_CS 3
#define OLED_RST 4
#endif
U8G2_SSD1309_128X64_NONAME2_1_4W_HW_SPI u8g2(/* rotation=*/U8G2_R0, /* cs=*/ OLED_CS, /* dc=*/ OLED_DC,/* reset=*/OLED_RST);
void setup(void) {
u8g2.begin();
u8g2.setFontPosTop();
}
void loop(void) {
u8g2.firstPage();
do
{
u8g2.clearBuffer(); // clear the internal memory
u8g2.setFont(u8g2_font_t0_17b_tr ); // choose a suitable font
u8g2.drawStr(0,5,"Hello World!"); // write something to the internal memory
u8g2.sendBuffer(); // transfer internal memory to the display
} while( u8g2.nextPage() );
delay(2000);
}
Result:
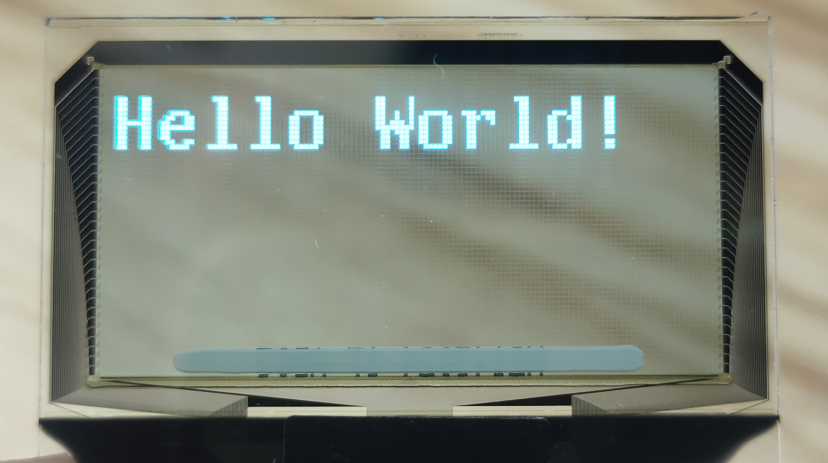
Sample Code 7-Language.ino
/*!
* @file Language.ino
* @brief Display multiple languages in U8G2
* @n A demo for displaying “hello world!” in Chinese, English, Japanese
* @n U8G2 Font GitHub Link:https://github.com/olikraus/u8g2/wiki/fntlistall
*
* @copyright Copyright (c) 2010 DFRobot Co.Ltd (http://www.dfrobot.com)
* @licence The MIT License (MIT)
* @author [Ivey](Ivey.lu@dfrobot.com)
* @maintainer [Fary](feng.yang@dfrobot.com)
* @version V1.0
* @date 2019-10-15
* @url https://github.com/DFRobot/U8g2_Arduino
*/
#include <Arduino.h>
#include <U8g2lib.h>
#include <SPI.h>
/*
* Display hardware IIC interface constructor
*@param rotation:U8G2_R0 Not rotate, horizontally, draw direction from left to right
U8G2_R1 Rotate clockwise 90 degrees, drawing direction from top to bottom
U8G2_R2 Rotate 180 degrees clockwise, drawing in right-to-left directions
U8G2_R3 Rotate clockwise 270 degrees, drawing direction from bottom to top
U8G2_MIRROR Normal display of mirror content (v2.6.x version used above)
Note: U8G2_MIRROR need to be used with setFlipMode().
*@param reset:U8x8_PIN_NONE Indicates that the pin is empty and no reset pin is used
* Display hardware SPI interface constructor
*@param Just connect the CS pin (pins are optional)
*@param Just connect the DC pin (pins are optional)
*
*/
#if defined ARDUINO_SAM_ZERO
#define OLED_DC 7
#define OLED_CS 5
#define OLED_RST 6
/*ESP32 */
#elif defined(ESP32)
#define OLED_DC D2
#define OLED_CS D6
#define OLED_RST D3
/*ESP8266*/
#elif defined(ESP8266)
#define OLED_DC D4
#define OLED_CS D6
#define OLED_RST D5
/*AVR series board*/
#else
#define OLED_DC 2
#define OLED_CS 3
#define OLED_RST 4
#endif
U8G2_SSD1309_128X64_NONAME2_1_4W_HW_SPI u8g2(/* rotation=*/U8G2_R0, /* cs=*/ OLED_CS, /* dc=*/ OLED_DC,/* reset=*/OLED_RST);
void setup(void) {
u8g2.begin(); //init
u8g2.enableUTF8Print(); // Enable UTF8 support for Arduino print()function.
}
void loop(void)
{
/*@brief Set font direction of all strings setFontDirection(uint8_t dir)
*@param dir=0,rotate 0 degree
dir=1,rotate 90 degrees
dir=2,rotate 180 degrees
dir=3,rotate 270 degrees
*@param When completed font setting, re-set the cursor position to display normally. Refer to API description for more details.
*/
u8g2.setFontDirection(0);
/*
* firstPage Change the current page number position to 0
* Revise content in firstPage and nextPage, re-render everything every time
* This method consumes less ram space than sendBuffer
*/
u8g2.firstPage();
do {
/*
*The font takes up a lot of memory, so please use it with caution. Get your own Chinese encode for displaying only several fixed words.
*Display by drawXBM or use controller with larger memory
*Chinese Font:require a controller with larger memory than Leonardo
*Japanese Font:require a controller with larger memory than UNO
*Korean Font:Arduino INO files of the current version do not support for displaying Korean, but it can displayed properly on the Screen
*/
u8g2.setFont(u8g2_font_HelvetiPixelOutline_tr);
u8g2.setCursor(/* x=*/0, /* y=*/15); //Define the cursor of print function, any output of the print function will start at this position.
u8g2.print("Hello World!");
u8g2.setFont(u8g2_font_unifont_t_chinese2);
u8g2.setCursor(0, 35);
u8g2.print("你好世界"); // Chinese "Hello World"
u8g2.setFont(u8g2_font_b10_t_japanese1);
u8g2.setCursor(0, 50);
u8g2.print("こんにちは世界");
} while ( u8g2.nextPage() );
delay(1000);
}
Result:
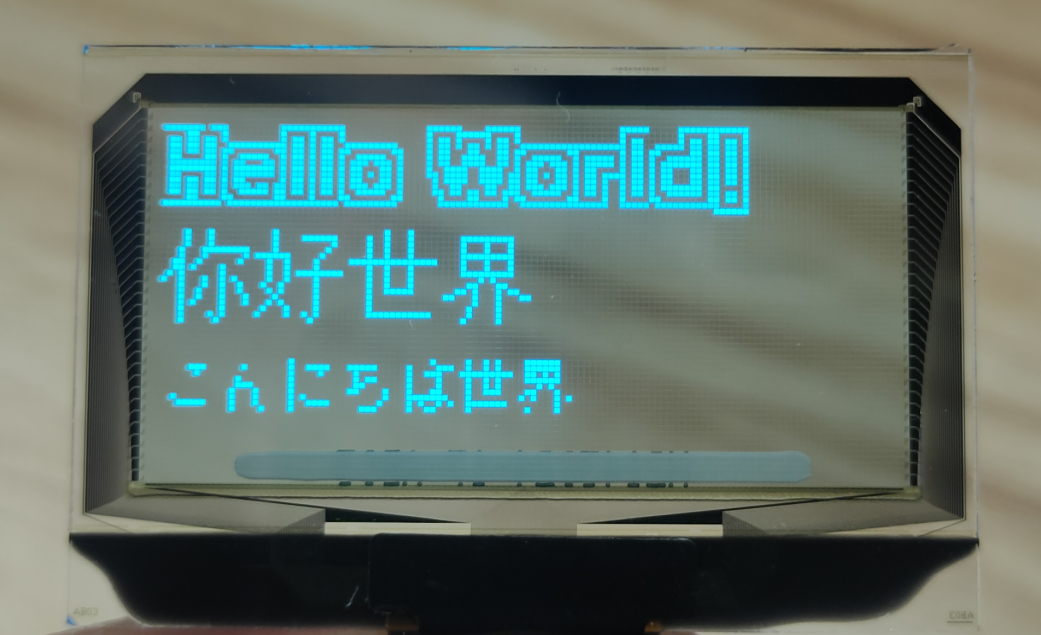
FAQ
For any questions, advice or cool ideas to share, please visit the DFRobot Forum.