Introduction
TF-NOVA is a sensor based on the Pulse Time-of-Flight (PToF) ranging principle. The laser diode at the transmitter emits pulsed laser signals collimated through a transmitting lens. The reflected signals from the target object pass through the receiving lens and are detected by the receiver’s photodetector. Internal circuitry computes the time difference between emitted and reflected signals, enabling distance calculation between the LiDAR and the target object based on the speed of light.
Line-Shaped Detection Area
TF-NOVA features a unique detection zone of 14° (horizontal) × 1° (vertical). It detects a 25 × 1.7 cm² area at distances beyond 1m, equivalent to the area of two flatly placed chopsticks.
Compact Size, Easy Integration
With ultra-compact dimensions of 26.5mm × 21.05mm × 12mm (~7 cm³ volume), the device is smaller than an AA battery. Its slim profile allows seamless integration into space-constrained, lightweight hardware architectures, offering exceptional flexibility for system design.
Stable Outdoor Detection
TF-NOVA delivers reliable detection of 5cm black low-reflectivity obstacles within 2m, even under intense outdoor sunlight or low-light nighttime conditions, ensuring robust operational safety for devices.
Comprehensive Interfaces
Equipped with mainstream interfaces (UART, I2C, I/O), it supports both standalone and networked operation. Configurable I/O output mode significantly reduces data processing workloads.
Features
- Compact size, easy integration
- 14°×1° line-shaped detection area
- Stable indoor/outdoor measurement
- UART, I2C, I/O output modes
Applications
- Automatic door collision prevention
- Robot obstacle avoidance
- Shuttle vehicle obstacle detection
- Parking space detection
- Focus assistance
Specifications
- Performance Parameter
- Measurement Range:
≥14m@90%reflectivity,0Klux; ≥13m@10%reflectivity, 0Klux;
≥7m@90%reflectivity,100Klux; ≥4m@10%reflectivity, 100Klux - Blind Zone: 0.1m
- Accuracy: ±5cm @ 0.1-4m
- Precision: <1cm (1σ) @ 0.1-4m
- Distance Resolution: 1cm
- Frame Rate: 1-900Hz (default: 100Hz)
- Optical Performance
- Light Source: VCSEL
- Central Wavelength: 905nm
- Laser FOV: 14°×1°
- Laser Safety: Class 1 Eye-safe [EN60825]
- Mechanical & Electrical
- Avg Power Consumption: <500mW
- Inrush Current: <850mA
- Startup Time: <1s
- Operating Voltage: DC 5V ±5%
- Operating Temperature: -25℃ ~ +70℃
- Storage Temperature: -30℃ ~ +80℃
- Dimensions: 26.5×21.05×12.0mm
- Weight: <5g
- Hardware Interface: 1.25mm-5Pin
- Front Window Protection: IP65
Board Overview
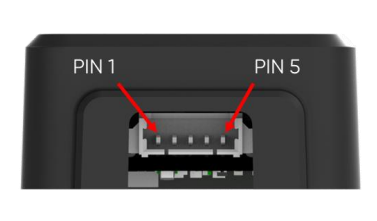
Num | Label | Description |
---|---|---|
PIN1 | Red line | 5V |
PIN2 | Black line | GND |
PIN3 | Yellow line | TXD(3.3V)/SCL |
PIN4 | Green line | RXD(3.3V)/SDA |
PIN5 | Blue line | IO |
TF-NOVA switches the output mode through instructions and registers. The default is UART output. For detailed instructions, please refer to the user manual.
Dimensional Drawing
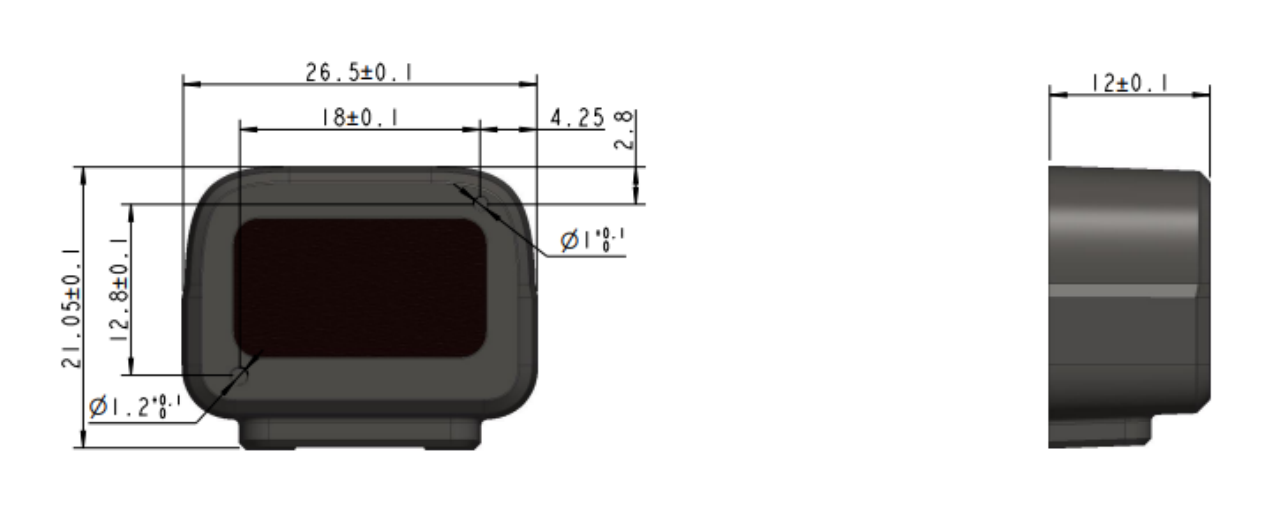
Tutorial
Requirements
- Hardware
- DFRduino UNO R3 (or similar) x 1
- Gravity: IO Expansion Shield for Arduino V7.1 x1
- TF-NOVA Line Laser LiDAR Sensor x1
- 1.25mm-to-2.54mm Dupont Cable (20cm) x1
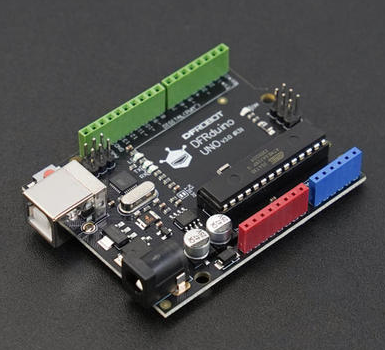
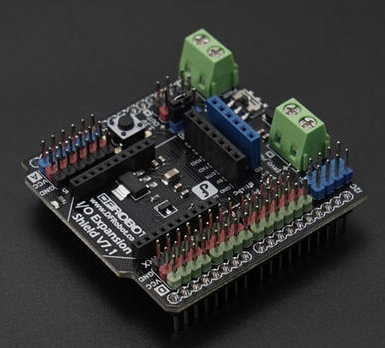
- Software
- Arduino IDE
- Download the DFRobot_TFmini library, which is a general library for TF series single-point LiDAR.
UART Mode Connection Diagram
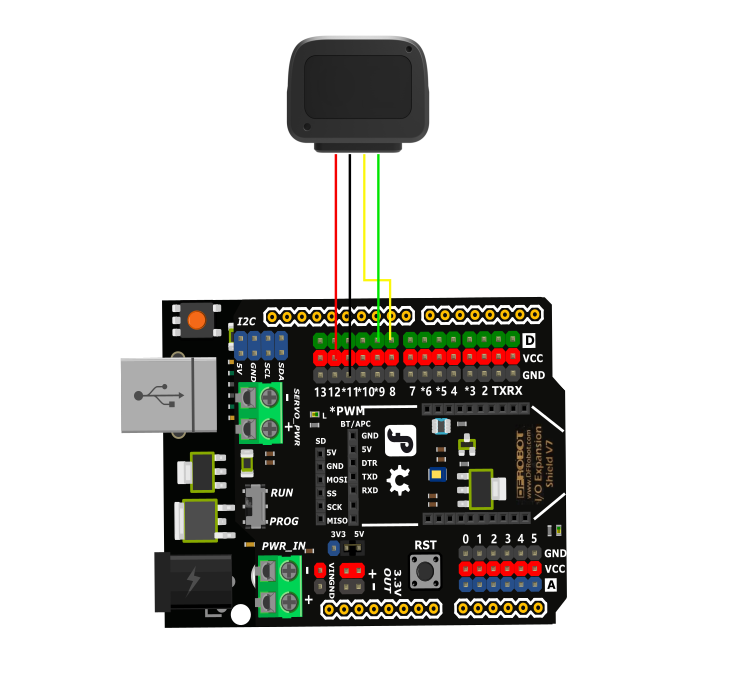
Sample Code
#include <DFRobot_TFmini.h>
SoftwareSerial mySerial(8, 9); // RX, TX
DFRobot_TFmini TFmini;
char COM[6] = { 0x5A, 0x06, 0x0A, 0x01, 0x01, 0x6C }; //I2C mode command
char COM1[4] = { 0x5A, 0x04, 0x11, 0x6F }; //Save setup command
uint16_t distance, strength;
void setup() {
Serial.begin(9600);
TFmini.begin(mySerial);
/*
* Send command, open when switching to I2C output mode
*/
// mySerial.print(COM);
// delay(200);
// mySerial.print(COM1);
}
void loop() {
if (TFmini.measure()) {
distance = TFmini.getDistance();
strength = TFmini.getStrength();
Serial.print("Distance = ");
Serial.print(distance);
Serial.println("cm");
Serial.print("Strength = ");
Serial.println(strength);
delay(100);
}
delay(100);
}
Expected Results
Print the collected distance value and signal strength value.
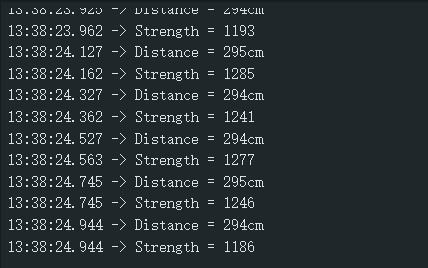
I2C Mode Connection Diagram
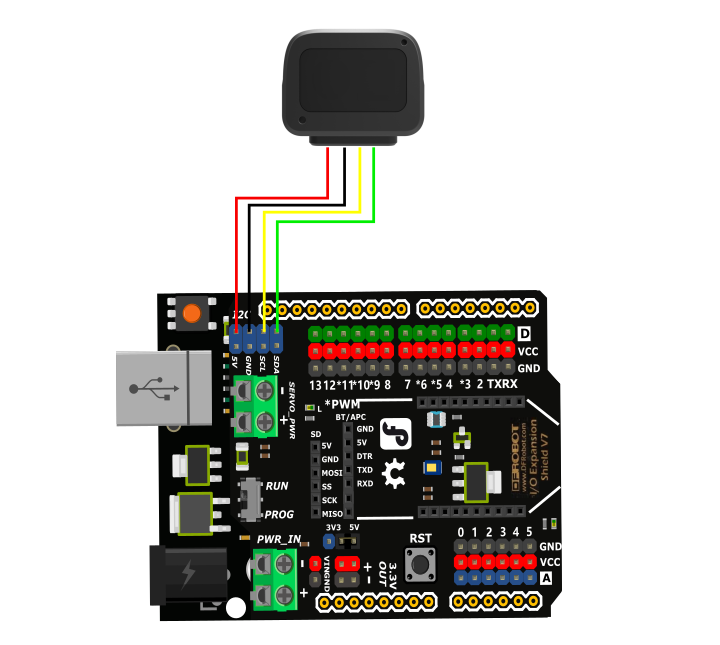
Sample Code
#include <Wire.h> //I2C library
#define deviceaddress 0x10
uint16_t data;
uint8_t COM[4] = { 0 };
uint8_t COM1[1] = { 0x00 }; //Serial port mode
uint8_t COM2[2] = { 0x01, 0x02 }; //Save, restart
uint8_t COM3[1] = { 0x64 }; //Frame rate value, default 0x64 (100hz)
uint8_t COM4[1] = { 0 };
void i2c_writeN(uint8_t registerAddress, uint8_t *buf, size_t len) {
Wire.beginTransmission(deviceaddress);
Wire.write(registerAddress);
Wire.write(buf, len);
Wire.endTransmission();
}
int16_t i2c_readN(uint8_t registerAddress, uint8_t *buf, size_t len) {
uint8_t i = 0;
Wire.beginTransmission(deviceaddress);
Wire.write(registerAddress);
if (Wire.endTransmission(false) != 0) {
return -1;
}
Wire.requestFrom(deviceaddress, len);
delay(100);
while (Wire.available()) {
buf[i++] = Wire.read();
}
return i;
}
void setup() {
Wire.begin();
Serial.begin(9600);
/*
* Write to register, open when you need to modify the frame rate
*/
// i2c_writeN(0x26, COM3, 1);
/*
* Write register, open when switching to UART mode
*/
// i2c_writeN(0x1E, COM1, 1);
// delay(200);
// i2c_writeN(0x20, COM2, 2);
}
void loop() {
i2c_readN(0x00, COM, 2);
data = COM[1] << 8 | COM[0];
i2c_readN(0x26, COM4, 1);
Serial.print("Distance =");
Serial.print(data);
Serial.print(" cm");
Serial.print(" FPS =");
Serial.print(COM4[0]);
Serial.println(" hz");
delay(100);
}
Expected Results
Print the collected distance value and frame rate value.
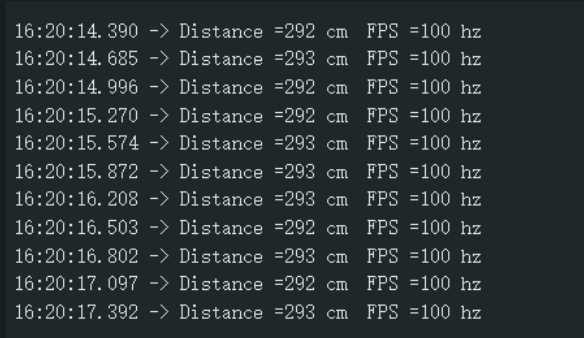
FAQ
- UART and I2C output modes are switched through instructions or registers
- TF-NOVA operates at 5V, and does not work at 3.3V